How to Get Parent in JavaScript
-
Get Parent With
parentNode
andparentElement
Properties -
Use the
parentNode
Property to Get the Parent -
Use the
parentElement
Property to Get Parent
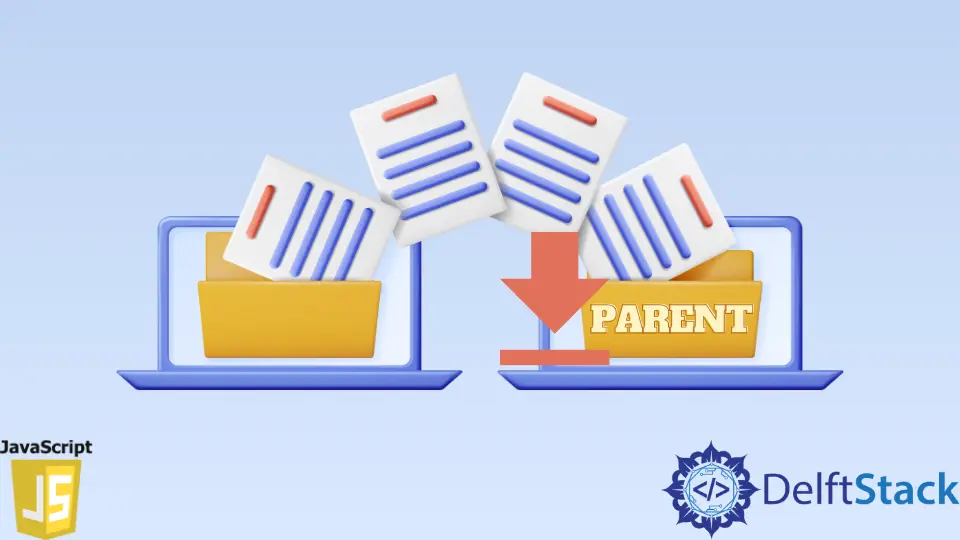
In JavaScript, the properties to get the name and detail of the parent element are parentNode
and parentElement
. These two properties play an identical role in defining the parent node, but their performance is slightly different.
The parentElement
is a read-only property that only focuses on the result of the parent element of a specified structure. On the contrary, parentNode
is also a read-only property, drawing out the object node representing one of the document tree nodes.
Get Parent With parentNode
and parentElement
Properties
In this example, we’ll examine the behavior of the two properties to get the parent of an HTML structure. If we define the difference, we will have one exception to display.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<script>
console.log(document.documentElement.parentNode);
console.log(document.documentElement.parentElement);
</script>
</body>
</html>
Output:
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<script>
console.log(document.documentElement.parentNode.nodeName);
console.log(document.documentElement.parentElement.nodeName);
</script>
</body>
</html>
Output:
As you can see, the parentNode
property has the #documenet
as its output node, but the parentElement
gives a null
. Also, when we tried to extract the node name, we received a TypeError
for parentElement
.
Use the parentNode
Property to Get the Parent
Let’s have an instance that will show that a child’s instance can be used to get its immediate parent from the hierarchy tree. We will take a nested div
structure and take the last stated div element as a child.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
</head>
<body>
<div id="parent">
<div id="p2">
<div id="child"></div>
</div>
</div>
<script>
var ch=document.getElementById("child");
console.log(ch.parentNode.nodeName);
console.log(ch.parentNode);
</script>
</body>
</html>
Output:
We have the node name as well as the node itself for demonstration. The selected parent was the one with the id-p2
.
Use the parentElement
Property to Get Parent
This example will get the immediate parent element of our selected child elements’ instance.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
</head>
<body>
<ul>
<li id="child"></li>
</ul>
<script>
var ch=document.getElementById("child");
console.log(ch.parentElement.nodeName);
</script>
</body>
</html>
Output: