How to Get Page Title in JavaScript
-
Use the
document.title
Property to Get Page Title in JavaScript -
Use
getElementsByTagName
to Get Page Title in JavaScript
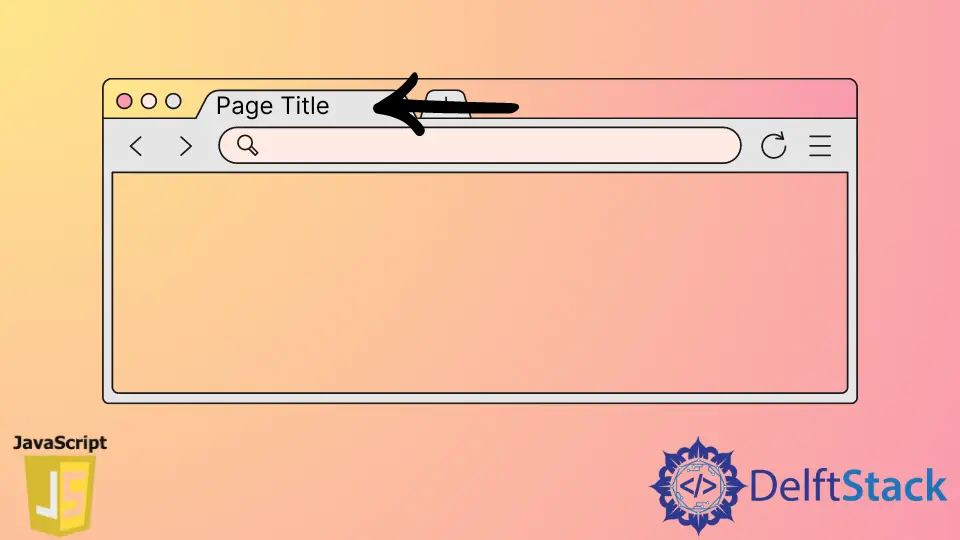
A page title gives a webpage a unique identification. This title can be grabbed or manipulated by some JavaScript properties.
The reason behind performing this task is to get notified about the page. In the following examples, we will see two instances that cover how to get the page title.
The first will focus on the document.title
property that can fetch and manipulate the title name. And secondly, we will see if we can get the title by making an instance of the TagName
.
Use the document.title
Property to Get Page Title in JavaScript
We will see how the document.title
property gets the title in this instance. If we do not set any new title for the page, the property will fetch the predefined title by default.
Here, the document.title
would return Test
, but as we set the value to Title Changed
, that is the new title.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<script>
document.title = "Title Changed"
console.log(document.title);
</script>
</body>
</html>
Output:
Use getElementsByTagName
to Get Page Title in JavaScript
In the case of dealing with the TagName
, we will take an instance of it and try to fetch its content, aka the title with the innerHTML
property.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Another Test</title>
</head>
<body>
<script>
var title = document.getElementsByTagName("title")[0].innerHTML;
console.log(title)
</script>
</body>
</html>
Output: