How to Get HTML From URL in JavaScript
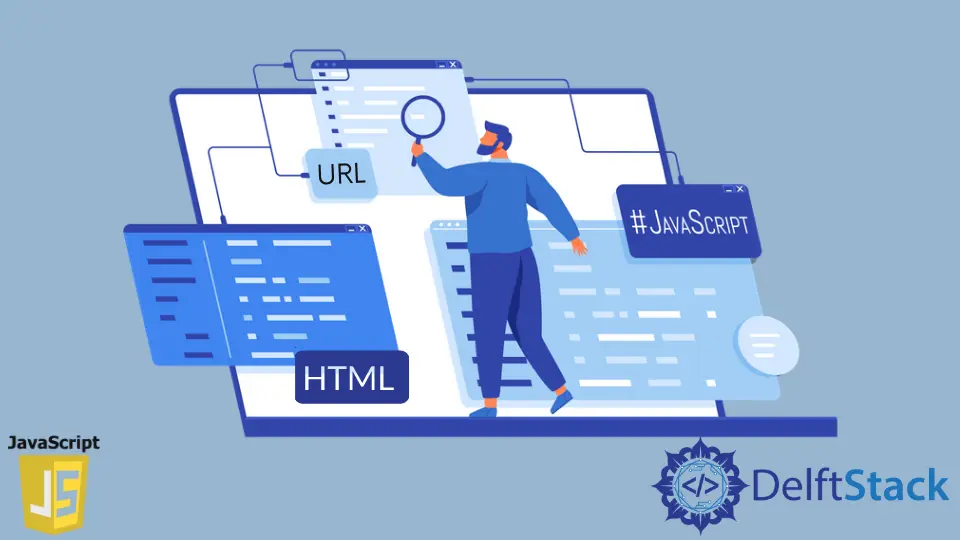
One can easily see the web page’s source code using browser dev tools.
But the interesting feature that JavaScript provides us is that we can get the source code of a different webpage on our page without having to visit that page. This post shows various methods of achieving this.
Use XMLHttpRequest()
to Get HTML
Code With a URL
XML HTTP Request (XHR) mainly serves to retrieve data from a URL without refreshing the page. So they can be used to get the HTML code from a different page.
function makeHttpObject() {
if ('XMLHttpRequest' in window)
return new XMLHttpRequest();
else if ('ActiveXObject' in window)
return new ActiveXObject('Msxml2.XMLHTTP');
}
var request = makeHttpObject();
request.open('GET', '/', true);
request.send(null);
request.onreadystatechange = function() {
if (request.readyState == 4) console.log(request.responseText);
};
In the above example, we first make the HTTP object an HTTP request.
Then we initialize and send the get request using open()
and send()
methods. We print the HTML code when the response becomes available.
Use jQuery
to Get HTML
Code With a URL
jQuery.ajax()
is used to perform asynchronous HTTP requests. It takes as an argument the URL
to send requests and settings
(a set of key-value pairs).
$.ajax({
url: '/',
success: function(data) {
console.log(data);
}
});
In the above example, we pass the URL
for the HTTP request, and if the request is a success, we print the data returned (i.e., the HTML code for the webpage).
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn