How to Continue the forEach Loop in JavaScript
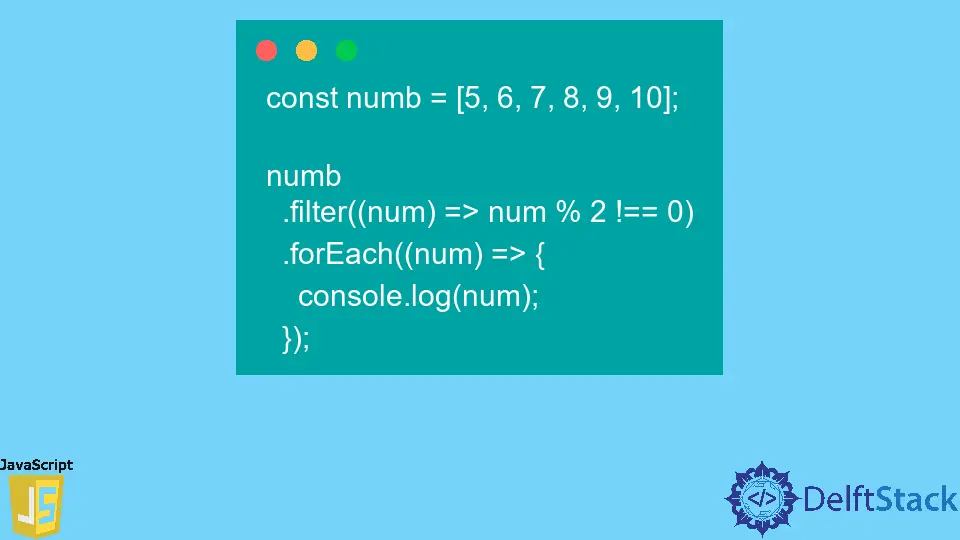
This tutorial will teach you how to continue the forEach
loop in JavaScript.
Continue the forEach
Loop in JavaScript
The forEach
loop is a JavaScript array method that performs a custom callback function on every item in an array. Only on the array can you utilize the forEach
loop.
Let’s start with a forEach
loop example:
Assume you have a numbers array with 5 to 10 numbers. How will you print the value using the forEach
loop?
See the demonstration below:
const numb = [5, 6, 7, 8, 9, 10];
numb.forEach((num) => {
console.log(num);
});
Output:
5,6,7,8,9,10
Assume you want to do something else; you want to skip any items in your numb
array that are even. How will you accomplish this?
In that loop, you append continue
. However, a problem will arise if you utilize continue
in a forEach
loop.
Let’s start with the problem:
const numb = [5, 6, 7, 8, 9, 10]
numb.forEach(num => {
if (num % 2 === 0) {
continue;
}
})
Output:
Uncaught SyntaxError: Illegal continue statement: no surrounding iteration statement
As you can see, a continue
statement inside a JavaScript forEach
loop results in an Uncaught SyntaxError
. Hence the return
statement is the better alternative.
We got a syntax error because the forEach
loop behaves more like a function than a loop. That is why you are unable to continue performing on it.
However, if you must use continue
in a forEach
loop, there is an option. A return
may be used to exit the forEach
loop.
Look at the code below:
const numb = [5, 6, 7, 8, 9, 10];
numb.forEach((num) => {
if (num % 2 === 0) {
return;
}
console.log(num);
});
Output:
5,7,9
Though return
may be used to get the necessary output, there is a more efficient method. You can easily remove the undesired values using the filter()
function.
Example:
const numb = [5, 6, 7, 8, 9, 10];
numb.filter((num) => num % 2 !== 0).forEach((num) => {
console.log(num);
});
Output:
5,7,9
Conclusion
To conclude this article, you cannot use a continue
statement directly inside a forEach
loop. Instead, the return
statement is the ideal solution.
If you cannot utilize the return
statement, you must substitute your forEach
loop with a for
or while
loop to use the continue
statement.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn