fillRect() Function in JavaScript
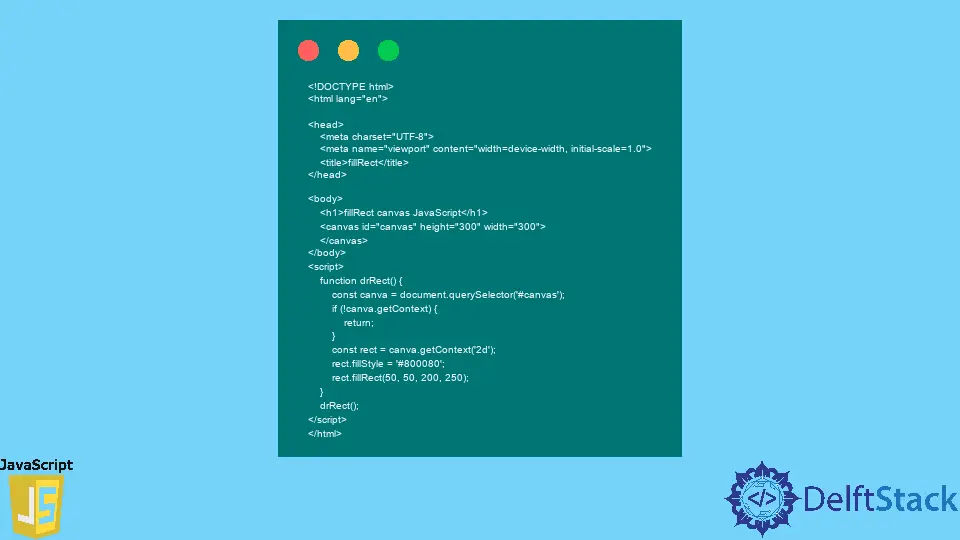
In this article, you’ll learn how to create rectangles with a defined width and height on a canvas using the JavaScript fillRect()
function.
Use the fillRect()
Function in JavaScript
The HTML canvas’ fillRect()
function produces a filled rectangle on the web page. Black
is the default color. Using JavaScript, you can draw pictures on a web page using the canvas
element.
Every canvas contains two components that indicate its height and width, height and width, respectively.
Syntax:
obj.fillRect(a, b, width, height);
The a
is the x-axis position of the Rectangle’s beginning point, and b
is the y-axis position of the Rectangle’s beginning point. The width
is the width of the rectangles, which can be both positive and negative, where the positive values are shown on the right, whereas negative values are shown on the left.
The height
is the height of the Rectangle, and it can also be either positive or negative. Positive values are decreasing while negative values are increasing.
Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>fillRect</title>
</head>
<body>
<h1>fillRect canvas JavaScript</h1>
<canvas id="canvas" height="300" width="300">
</canvas>
</body>
<script>
function drRect() {
const canva = document.querySelector('#canvas');
if (!canva.getContext) {
return;
}
const rect = canva.getContext('2d');
rect.fillStyle = '#800080';
rect.fillRect(50, 50, 200, 250);
}
drRect();
</script>
</html>
Use the document.querySelector()
method to choose the canvas element. Determine if the browser supports the canvas API.
Obtain the 2D drawing context object. Change the fill style to #800080
, purple
color and use the fillRect()
function to draw the Rectangle.
The Rectangle has a width of 200 pixels and a height of 250 pixels, starting at (50, 50).
Output:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn