How to Create Fibonacci in JavaScript
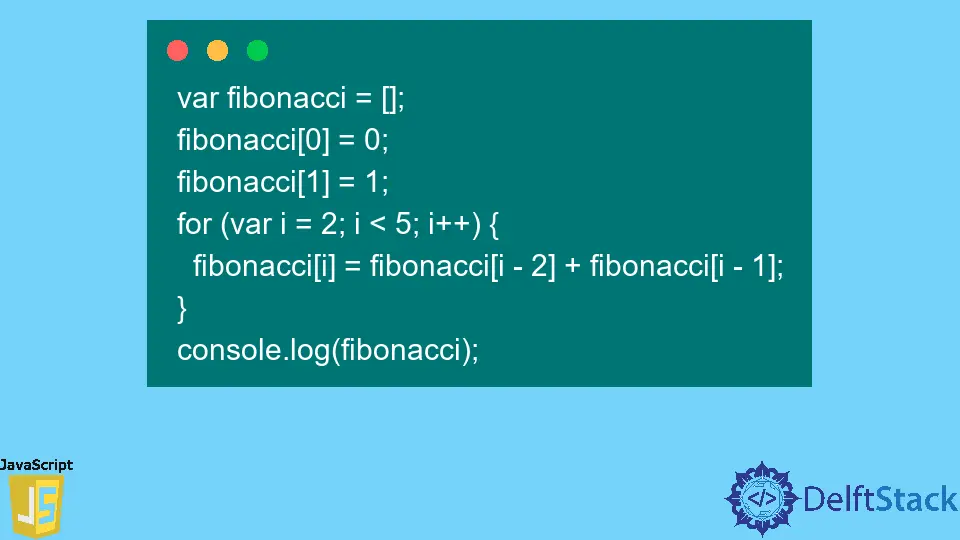
This tutorial will discuss how to generate a Fibonacci sequence using a loop in JavaScript.
Generate a Fibonacci Sequence Using a Loop in JavaScript
In Fibonacci sequence, the first and second value is 0 and 1, and all the other values will be calculated based on the previous two values. For example, the third value of the Fibonacci sequence is the sum of the first two values and so on.
To generate the Fibonacci Sequence in JavaScript, we have to define the first two values, and then we will use a loop that will generate the rest of the values by adding two previous values of the sequence. For example, let’s generate the first five values of the Fibonacci sequence in JavaScript. See the code below.
var fibonacci = [];
fibonacci[0] = 0;
fibonacci[1] = 1;
for (var i = 2; i < 5; i++) {
fibonacci[i] = fibonacci[i - 2] + fibonacci[i - 1];
}
console.log(fibonacci);
Output:
(5) [0, 1, 1, 2, 3]
As you can see in the output, the first five values of the Fibonacci sequence have been generated. We can also make a function using the above code, so we only have to give the number of values we want to generate to the function that will generate the Fibonacci sequence. For example, let’s make the function to generate the Fibonacci sequence given the number of values and test it to generate 10 values and show the result on the console using the console.log()
function. See the code below.
function GenerateFibonacci(number) {
var fibonacci = [];
fibonacci[0] = 0;
fibonacci[1] = 1;
for (var i = 2; i < number; i++) {
fibonacci[i] = fibonacci[i - 2] + fibonacci[i - 1];
}
return fibonacci;
}
var f = GenerateFibonacci(10);
console.log(f);
Output:
(10) [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
As you can see in the output, the first ten values of the Fibonacci sequence have been generated. You can use this function to generate as many values of the Fibonacci sequence as you want.