How to Implement Fade-In Div in JavaScript
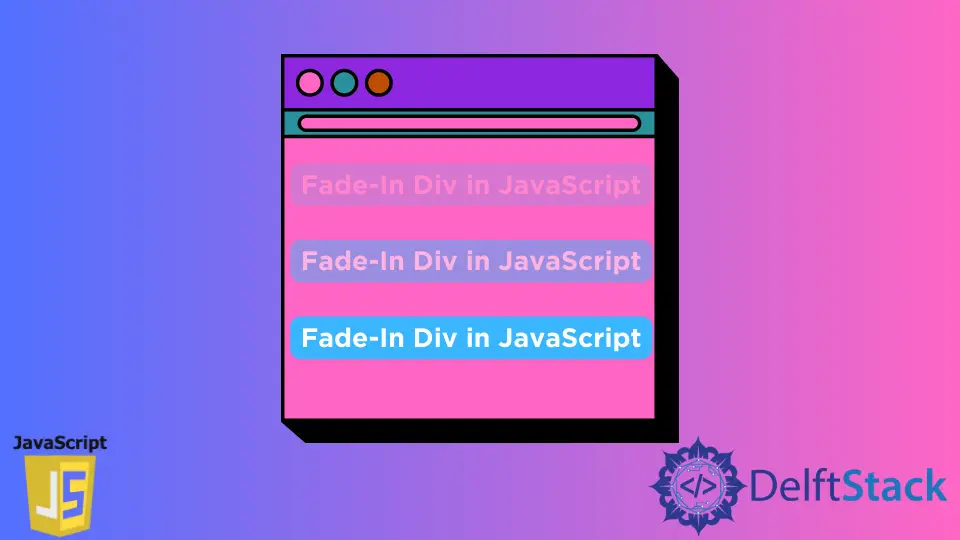
CSS animations can help you animate transitions from one CSS style place to another.
Animations encompass components, a style that describes the CSS animation, and a sequence of keyframes that imply the beginning and finishing states of the animation style and all states in between reference points.
In today’s post, we’ll learn to fade in and out div using pure JavaScript.
Fade-In Div in JavaScript
A CSS fade
transition is an effect where an element, e.g., text, image, or background, gradually appears or disappears on the page. To create these effects, use the transition or animation property in CSS.
Steps to Create a Fade-In Function in JavaScript
- Initialize an
opacity
variable with0.1
. - Set the element’s
display
property toblock
to make it visible as an individual box. - Define the timer, and trigger it every
500
milliseconds to update the opacity by10%
. - Once the
opacity
is set, update the filter attribute of the element to the new opacity. - Clear the interval and stop the timer once the element’s opacity reaches
1
.
Let’s create a div with Hello World!
text. Each time the page loads, this animation plays, and the page appears to fade in.
The fade time can be set in the set interval.
<div id="fadeEl" class="fadeElClass"
onclick="fadeIn()">
Hello World!
</div>
.fadeElClass {
width: 200px;
height: 20px;
text-align: center;
background: red;
}
function fadeIn() {
const element = document.getElementById('fadeEl');
console.log(element.style);
// Initilize the opacity with 0.1
let initOpacity = 0.1;
element.style.display = 'block';
// Update the opacity with 0.1 every 10 milliseconds
const timer = setInterval(function() {
if (initOpacity >= 1) {
clearInterval(timer);
}
element.style.opacity = initOpacity;
element.style.filter = 'alpha(opacity=' + initOpacity * 100 + ')';
initOpacity += initOpacity * 0.1;
}, 500);
}
fadeIn()
Try to run the above code in any browser; it will fade in hello world
text from 0.1 to 1 opacity.
Output:
Before Fade-in:
After Fade-in:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn