How to Extend Class in JavaScript
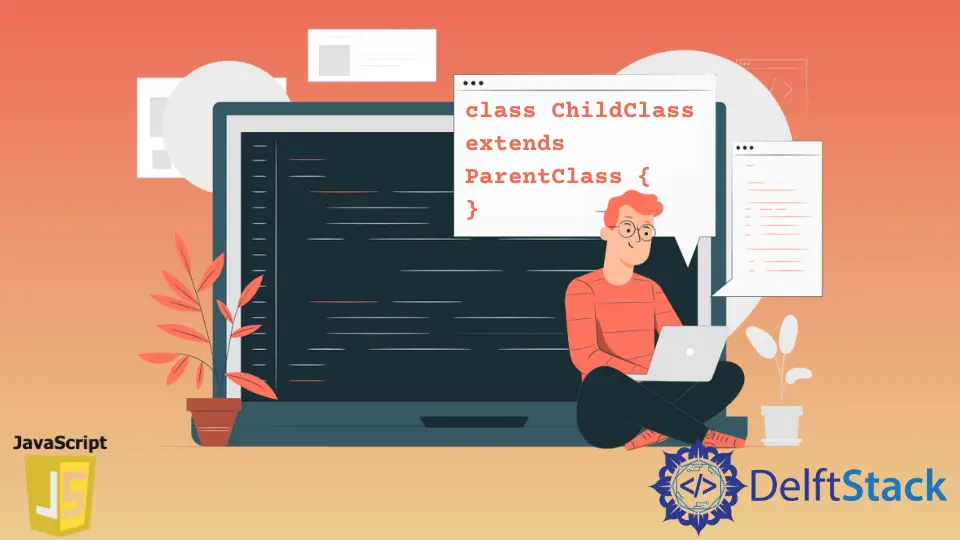
Classes are a template or blueprint for creating objects. You can encapsulate data with code to work with that data.
Classes in JS are based on prototypes, but they also have syntax and semantics that are not shared with ES5’s class semantics.
In today’s post, we’ll learn to extend the class in JavaScript.
Extend Class in JavaScript Using the extends
Keyword
The extends
keyword can derive custom classes and built-in objects from the main classes. It is used in class expressions or class declarations to create a class that is a child of another.
Syntax:
class ChildClass extends ParentClass { /* Logic goes here */
}
You can find more information about extends
in the documentation here.
For example, we have a ParentClass
consisting of the parent class’s name property and a counter that stores the number value. ParentClass
also contains a static method that displays the current instance of the parent class’s name property.
ChildClass
extends the properties of ParentClass
. By extending the parent class, the child class can access every parent class property.
The reserved keyword super
can execute super constructor calls and provides access to the parent methods.
Example:
class ParentClass {
constructor(counter) {
this.name = 'ParentClass';
this.counter = counter;
}
sayClassName() {
console.log('Hi, I am a ', this.name + '.');
}
}
const parent = new ParentClass(300);
parent.sayClassName();
console.log('The counter of this parent class is ' + parent.counter);
class ChildClass extends ParentClass {
constructor(counter) {
super(counter);
this.name = 'ChildClass';
}
get counter() {
return this.counter;
}
set counter(value) {
this.childCounter = value;
}
}
const child = new ChildClass(5);
child.sayClassName();
console.log('The counter of this parent class is ' + child.childCounter);
We have created a parent class instance that sets the counter to 300. As soon as the child class is generated, it updates the counter to 5.
The child class does not have counter property. It is inherited from the parent class.
Output:
"Hi, I am a ", "ParentClass."
"The counter of this parent class is 300"
"Hi, I am a ", "ChildClass."
"The counter of this parent class is 5"
You may also access the complete code of the example here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn