Exponents in JavaScript
- Understanding Exponents
- Using Math.pow() Function
- Using Exponentiation Operator (**)
- Creating a Custom Exponent Function
- Conclusion
- FAQ
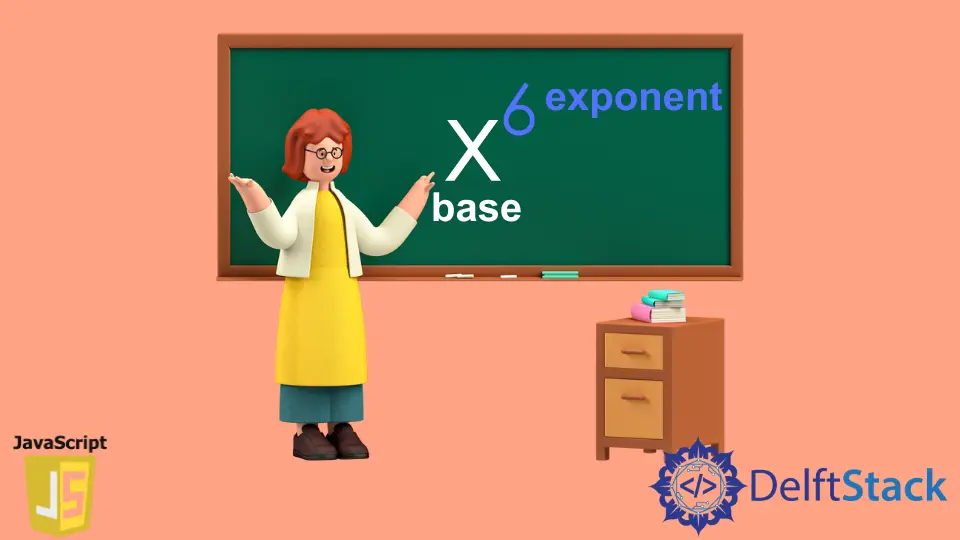
JavaScript is a versatile programming language that allows developers to perform a wide range of mathematical operations, including calculating exponents. Understanding how to work with exponents is crucial for various applications, from simple calculations to complex algorithms.
In this tutorial, we will explore different methods to calculate exponents in JavaScript, focusing on how to raise a number to a specific power. Whether you’re a beginner or looking to refresh your knowledge, this guide will equip you with the necessary skills to handle exponents effectively. Let’s dive into the world of exponents in JavaScript and discover how to harness their power in your coding projects!
Understanding Exponents
Before we jump into the code, let’s clarify what exponents are. In mathematical terms, an exponent refers to the number of times a base is multiplied by itself. For example, in the expression (2^3), 2 is the base, and 3 is the exponent, which means (2 \times 2 \times 2 = 8). In JavaScript, we can calculate exponents using several methods, including the Math.pow() function, the exponentiation operator (**), and custom functions.
Using Math.pow() Function
One of the most straightforward ways to calculate exponents in JavaScript is by using the built-in Math.pow() function. This function takes two arguments: the base and the exponent. Here’s how you can use it:
javascriptCopylet base = 2;
let exponent = 3;
let result = Math.pow(base, exponent);
console.log(result);
Output:
textCopy8
In this example, we defined a base of 2 and an exponent of 3. By passing these values to the Math.pow() function, we calculated (2^3), which equals 8. The Math.pow() function is widely used due to its simplicity and readability. It is especially useful for cases where you need to raise numbers to powers dynamically, such as in loops or when processing user input.
Using Exponentiation Operator (**)
Another modern and concise way to calculate exponents in JavaScript is by using the exponentiation operator (**). This operator is a part of the ECMAScript 2016 (ES7) specification and offers a more intuitive syntax for exponentiation. Here’s an example:
javascriptCopylet base = 2;
let exponent = 3;
let result = base ** exponent;
console.log(result);
Output:
textCopy8
In this code snippet, we again have a base of 2 and an exponent of 3. The exponentiation operator (**), placed between the base and the exponent, computes (2^3) in a clean and straightforward manner. This method is particularly advantageous for developers who prefer a more concise syntax, and it enhances code readability.
Creating a Custom Exponent Function
If you want to have more control over the exponent calculation or to implement additional features, creating a custom function can be beneficial. Here’s how you can define a function to calculate exponents:
javascriptCopyfunction customPow(base, exponent) {
let result = 1;
for (let i = 0; i < exponent; i++) {
result *= base;
}
return result;
}
let base = 2;
let exponent = 3;
let result = customPow(base, exponent);
console.log(result);
Output:
textCopy8
In this example, we defined a function called customPow that takes a base and an exponent as parameters. Inside the function, we initialize a result variable to 1. We then use a for loop to multiply the base by itself for the number of times specified by the exponent. This method gives you a clear understanding of how exponentiation works under the hood. It’s also a great exercise for practicing loops and function creation in JavaScript.
Conclusion
In this tutorial, we explored various methods to calculate exponents in JavaScript. From using the built-in Math.pow() function to leveraging the modern exponentiation operator and even creating a custom function, you now have a solid foundation in handling exponents. Understanding these methods will not only enhance your coding skills but also empower you to tackle more complex mathematical problems in your projects. So go ahead, experiment with these techniques, and elevate your JavaScript programming to new heights!
FAQ
- What is an exponent in mathematics?
An exponent indicates how many times a number is multiplied by itself.
-
How do I calculate exponents in JavaScript?
You can use Math.pow(), the exponentiation operator (**), or create a custom function. -
Is the exponentiation operator supported in all browsers?
Most modern browsers support the exponentiation operator, but it is advisable to check compatibility for older versions. -
Can I use negative exponents in JavaScript?
Yes, negative exponents can be handled in JavaScript, and they represent the reciprocal of the base raised to the absolute value of the exponent. -
What is the difference between Math.pow() and the exponentiation operator?
Math.pow() is a function that takes two arguments, while the exponentiation operator is a more concise syntax for the same operation.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn