How to Exit JavaScript Function
-
Use
return
to Exit a Function in JavaScript -
Use
break
to Exit a Function in JavaScript -
Use
try...catch
to Exit a Function in JavaScript
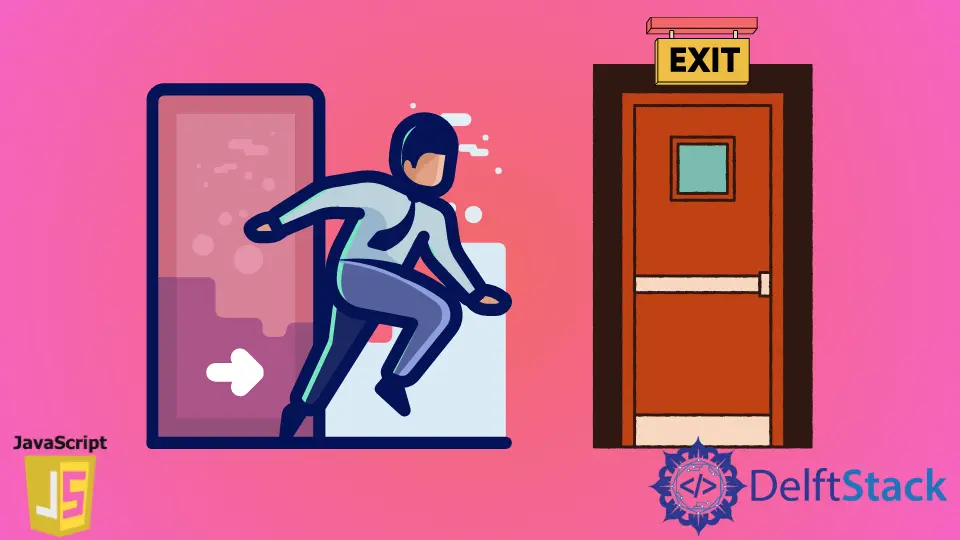
This tutorial explains how we can exit early from a function in JavaScript.
We often come across situations where we want to exit early from a function, like when a specific condition is satisfied. But JavaScript does not have an explicit function like other programming languages such as C++, PHP, etc. Different methods help us to exit early from a function.
There are three major ways that JavaScript explicitly provides us to exit a function early from its natural flow, namely return
, break
and try and catch
.
Use return
to Exit a Function in JavaScript
We can exit a function by using the return
statement when a specific condition is met. We can exit a function by using the return
statement alone or return a value from the function.
function divide(a, b) {
if (b == 0) {
return 'invalid b';
} else
return a / b;
}
console.log(divide(5, 2));
console.log(divide(5, 0));
Output:
2.5
invalid b
In this function, we first check if b
is 0
to rule out the case of invalid division because dividing a number by 0
returns infinity
, so we exit the function early by returning a string declaring that the value of b
is invalid. The statement performing division of a
and b
is never executed.
Use break
to Exit a Function in JavaScript
The break
is traditionally used to exit from a for
loop, but it can be used to exit from a function by using labels within the function.
const logIN = () => {
logIN: {console.log('I get logged in'); break logIN;
// nothing after this gets executed
console.log('I don\'t get logged in');}
};
logIN();
Output:
I get logged in
Here, we use the label logIN
and then break
out of the label
to exit the function earlier.
Use try...catch
to Exit a Function in JavaScript
We can use the try...catch
block to exit the function early by throwing an exception.
function myFunc() {
var a = 100;
try {
if (typeof (a) != 'string') throw (a + ' is not a string');
} catch (e) {
alert('Error: ' + e);
}
a++;
return a;
}
myFunc();
Output:
Error: 100 is not a string
We throw an exception to break out the normal flow, and the catch
block catches this exception, and then we exit the function leaving all other statements unexecuted.
All the major browsers support all these three ways.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn