The Excamation Operator in JavaScript
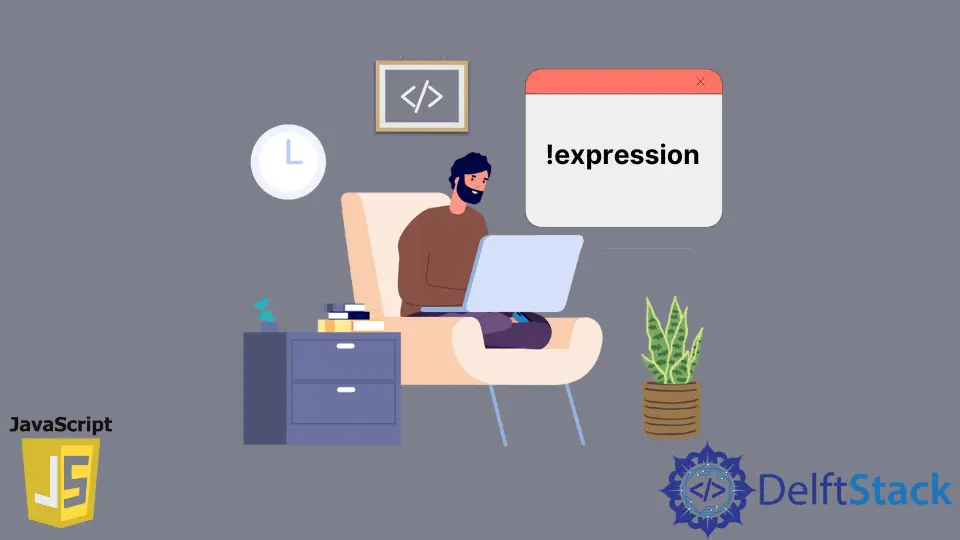
This tutorial introduces the behavior and use of the Logical Not(!
) operator in JavaScript.
The !
operator converts the operand to a boolean value and returns the inverse of the converted value.
Syntax
inverse = !expression
When we apply the !
operator to Falsy values, we get true
as a result. Falsy values includes – false
, 0
, -0
, 0n
, ""
, null
, undefined
, and NaN
.
When we apply the !
operator to the truthy value, we get false
as a result. Other than the mentioned falsy values, all values are truthy values.
Example 1
// boolean values
console.log('!true =>', !true); // returns false
console.log('!false =>', !false); // returns true
Output:
!true => false
!false => true
In the above code,
- Applyling the
!
operator to thetrue
value,false
is returned. - Applyling the
!
operator to thefalse
value,true
is returned.
Example 2
// string values
console.log('!(\'\') =>', !('')); // returns true
console.log('!(\'truthy_string\') => ', !('truthy_string')); // returns false
Output:
!('') => true
!('truthy_string') => false
In the above code,
- Applyling the
!
operator to the empty string(empty string represents falsy value),true
is returned. - Applyling the
!
operator to the non-empty string(non-empty string represents truthy value),false
is returned.
Example 3
// number values
console.log('!0 => ', !0); // returns true
console.log('!100 => ', !100); // returns false
Output:
!0 => true
!100 => false
In the above code,
- Applyling the
!
operator to the0
(0
represents falsy value),true
is returned. - Applyling the
!
operator to the100
(non-zero values representtruthy
value),false
is returned.
Example 4
console.log('!null => ', !null); // true
console.log('!undefined => ', !undefined); // true
console.log('!NaN => ', !NaN); // true
Output:
!null => true
!undefined => true
!NaN => true
We have applied the !
operator to the falsy values. For all the cases, we get true as a result.
Example 5
function returnsTruthyValue() {
return 100;
}
function returnsFalsyValue() {
return 0;
}
// apply ! to the return value
console.log('!returnsTruthyValue() =>', !returnsTruthyValue()); // false
console.log('!returnsFalsyValue() =>', !returnsFalsyValue()); // true
Output:
"!returnsTruthyValue() => false
"!returnsFalsyValue() => true
In the above code, we have created two functions.
returnsTruthyValue
function will return 100(truthy value).returnsFalsyValue
function will return 0(falsy value).
When we apply !
to the function call, the !
will be applied for the value returned by the function. !returnsTruthyValue()
will apply !
operator to the value returned by the returnsTruthyValue
function. So,
- Applyling
!
operator to thereturnsTruthyValue()
function will returnfalse
. - Applyling
!
operator to thereturnsFalsyValue()
function will returntrue
.
!!
Double Not in JavaScript
The !!
operator will return true
for truthy values and false
for falsy values. This operator is used to convert a value to a Boolean value.
Syntax
!!expression
The !!
will perform two operations.
- Convert the value of the expression to Boolean value and apply inverse to it.
- Again, the inverse is applied to the value.
Example
console.log('!!true =>', !!true) // true
console.log('!! 0 =>', !!0) // false
Output:
!!true => true
!! 0 => false
In the above code,
- Applyling
!!
operator totrue
, which will first converttrue
tofalse
and again convert thefalse
totrue
. - Applyling
!!
operator to0
, which will first convert0
to a boolean value(0
is converted asfalse
) and apply inverse to it(now the value will betrue
). Then one more time, the inverse is applied to the previously inversed value(now the value will befalse
).