JavaScript eval() Method Alternative
- Understanding the Risks of eval()
- Using Function Constructor
- Using JSON.parse for Object Literal Evaluation
- Using Template Literals for Dynamic Code
- Conclusion
- FAQ
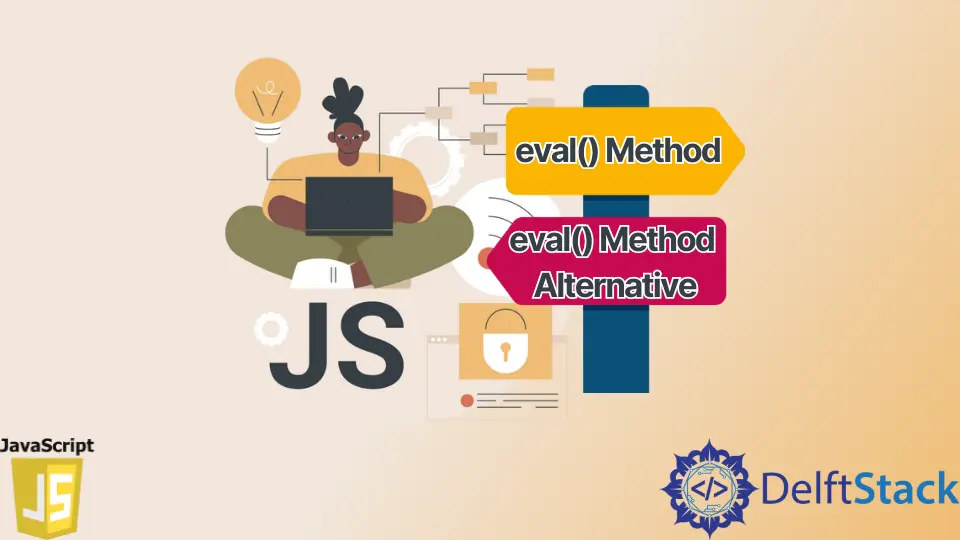
JavaScript’s eval()
method has long been a topic of debate among developers. While it allows for dynamic execution of code snippets, it poses significant security risks and performance issues.
In this article, we will explore alternatives to the eval()
method that can help you achieve similar functionality without compromising the integrity of your application. Whether you’re working with user-generated content or need to execute dynamic code, understanding these alternatives will enhance your coding practices. We will delve into safer methods that not only improve security but also maintain performance. Let’s dive into the world of JavaScript and discover how to replace the eval()
method effectively.
Understanding the Risks of eval()
Before we explore alternatives, it’s essential to understand why using eval()
can be problematic. The eval()
function executes a string as JavaScript code, which can lead to code injection vulnerabilities if not handled properly. This is particularly concerning when dealing with user input, as malicious users can inject harmful code that could compromise your application. Additionally, eval()
can slow down your code execution because it forces the JavaScript engine to recompile the code every time it runs. Therefore, finding a reliable alternative is crucial for writing secure and efficient JavaScript.
Using Function Constructor
One of the most common alternatives to eval()
is the Function constructor. This method allows you to create a new function from a string, providing a controlled environment to execute code. By using the Function constructor, you can avoid some of the risks associated with eval()
while still executing dynamic code.
Here’s how it works:
const dynamicCode = "return 2 + 2;";
const sumFunction = new Function(dynamicCode);
const result = sumFunction();
Output:
```text
4
In this code snippet, we define a string containing a simple arithmetic operation. We then create a new function using the Function constructor, passing the string as an argument. When we call sumFunction()
, it executes the code and returns the result. This method is safer than eval()
because it doesn’t have access to the local scope, reducing the risk of unintended side effects.
The Function constructor is particularly useful when you need to dynamically create functions based on user input or other variables. However, be cautious about the source of the strings you pass to it, as they can still pose security risks if not properly sanitized.
Using JSON.parse for Object Literal Evaluation
If your goal is to evaluate JSON data, using JSON.parse()
is a far safer alternative to eval()
. This method parses a JSON string and converts it into a JavaScript object. It’s a reliable way to handle data without the security concerns associated with executing arbitrary code.
Here’s a simple example:
const jsonString = '{"name": "John", "age": 30}';
const userObject = JSON.parse(jsonString);
Output:
```text
{name: "John", age: 30}
In this example, we have a JSON string representing a user object. By using JSON.parse()
, we convert this string into a JavaScript object. This method is not only safer but also faster because it directly interprets the JSON format without the overhead of executing code. It’s the go-to method for handling JSON data in JavaScript applications.
Using JSON.parse()
is particularly beneficial when working with APIs that return JSON data. It allows you to easily convert this data into usable JavaScript objects, enabling seamless integration with your application.
Using Template Literals for Dynamic Code
Another alternative to eval()
is using template literals, which allow you to embed expressions within string literals. This method is particularly useful for building dynamic strings without the need for code execution. Template literals can help you construct strings that include variables and expressions, making your code cleaner and more maintainable.
Here’s an example:
const a = 5;
const b = 10;
const resultString = `The sum of ${a} and ${b} is ${a + b}.`;
Output:
```text
The sum of 5 and 10 is 15.
In this code, we use template literals to create a string that describes the sum of two variables. This method avoids the need for dynamic code execution while still allowing for flexibility in string construction. Template literals are not only safer but also enhance code readability.
Using template literals is especially advantageous when generating dynamic content for web applications. By constructing strings in this way, you can easily include variables and expressions without compromising security.
Conclusion
In summary, while the eval()
method has its uses, it’s essential to consider safer alternatives that protect your application from potential vulnerabilities and performance issues. The Function constructor, JSON.parse(), and template literals offer effective ways to execute dynamic code, handle JSON data, and build strings without the risks associated with eval()
. By adopting these alternatives, you can write cleaner, more secure JavaScript code that enhances the overall quality of your applications.
FAQ
-
What is the main risk of using eval() in JavaScript?
The main risk of using eval() is that it can execute arbitrary code, which may lead to security vulnerabilities like code injection attacks. -
Can I still use eval() safely?
While it is possible to use eval() safely by sanitizing inputs, it is generally recommended to avoid it altogether in favor of safer alternatives. -
What is the Function constructor in JavaScript?
The Function constructor creates a new function from a string of code, allowing for dynamic execution without the risks associated with eval(). -
When should I use JSON.parse()?
You should use JSON.parse() when you need to convert a JSON string into a JavaScript object, especially when handling data from APIs. -
Are template literals a secure way to handle dynamic strings?
Yes, template literals are a secure and clean way to construct dynamic strings without executing code, making them safer than eval().
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn