How to Escape Backslash in JavaScript
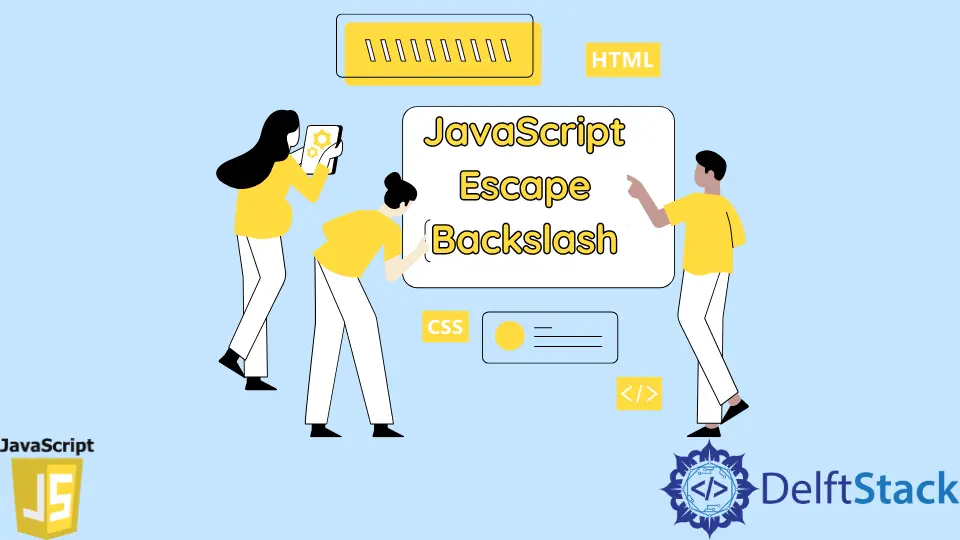
In today’s post, we’ll learn about escaping backslash in JavaScript.
Escape Backslash in JavaScript
Escape characters are the symbol used to start an escape command to operate. They are characters that can be interpreted differently than we intended.
JavaScript uses \
in front as an escape character.
The backslash \
is an escape character in JavaScript, just like many other languages. JavaScript tries to escape the next character when it encounters a backslash.
For example, \n
is a newline character (instead of a backslash followed by the letter n
). The backslash in JavaScript \
is reserved for use as an escape character.
To create a literal backslash, you must escape it. This means that \\
prints a single backslash and \\\\
prints two, etc.
let string = 'Hello World. Welcome to my \\ BlogPost \ ';
string = string.split('\\');
console.log(string);
We have defined the string with a backslash in the above example. We have split the string into two parts, separated by a backslash.
To escape the backslash in JavaScript, we have used the regex \\
.
The output of the above example is:
["Hello World. Welcome to my ", " BlogPost "]
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn