JavaScript Enums
Ammar Ali
Oct 12, 2023
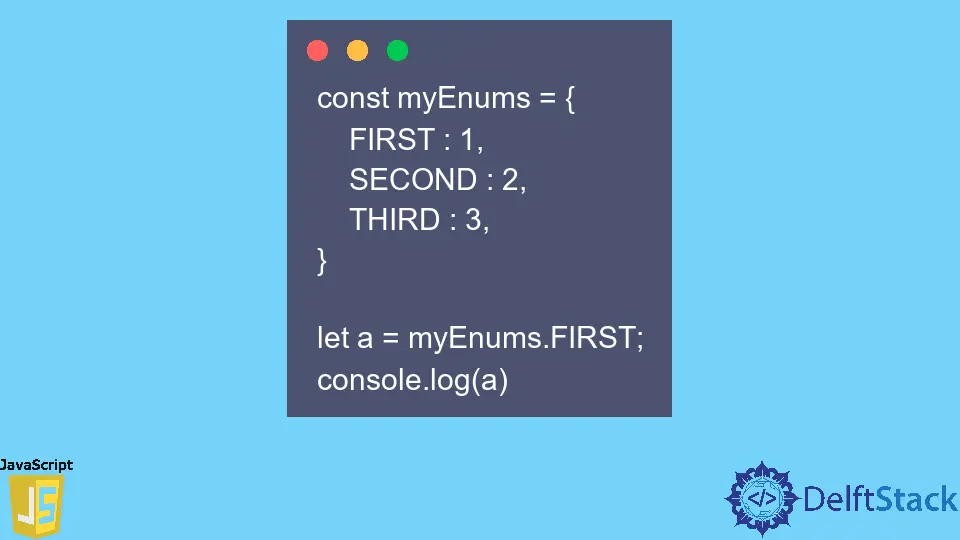
This tutorial will discuss how to define Enums
using curly brackets and upper case letters in JavaScript.
Define Enums
in JavaScript
Enums
, also known as enumerations, are used to define constant values in JavaScript. You can late use these values in your code. To define the Enums
, you can use the const
keyword and curly brackets. The name of the Enums
will be in upper case letters, and the value will be defined after a colon, and a comma will separate each Enum
from the other Enums
. For example, let’s define some Enums
and get their value by using the name of the Enum
and show it on the console. See the code below.
const myEnums = {
FIRST: 1,
SECOND: 2,
THIRD: 3,
}
let a = myEnums.FIRST;
console.log(a)
Output:
1
You can save any data type value inside the Enums
like string, integer, etc.