The Elvis Operator in JavaScript
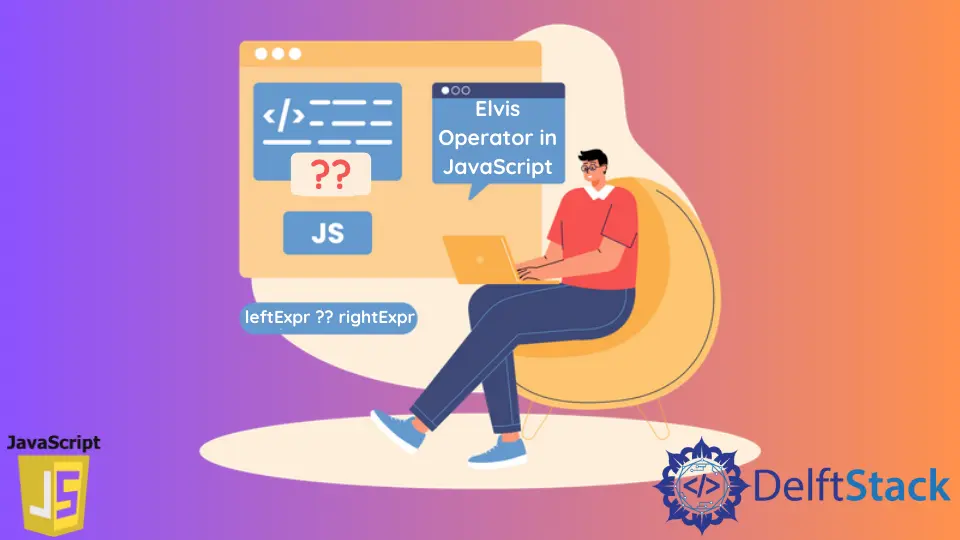
The Elvis Operator is a shorter version of the ternary operator. This operator is known as the nullish
coalescing operator in JavaScript, and it is represented using the ??
symbol.
It returns a default value only when the expression value is null
or undefined
.
Implement nullish
Coalescing Operator ??
in JavaScript
The nullish
coalescing operator ??
was introduced in the ECMAScript 2020 version of the JavaScript programming language.
Syntax:
leftExpr ?? rightExpr
The ??
operator will only return the rightExpr
(the expression that is present at the right of the ??
operator) only when the leftExpr
(the expression that is present at the left of the ??
operator) is either null
or undefined
.
Code:
let myObj = {name: {firstname: 'Adam', lastname: 'Smith'}};
let val = myObj.name ?? 'Default Value';
console.log(val);
Here, we have created an object called myObj
that contains a property called name
containing an entire object as a value. Then we are using the ??
operator.
To the left of the ??
operator, we have the myObj.name
expression, whereas to the right, we have the Default value
expression. The right expression, i.e., the Default Value, will only be assigned to the val
variable if the myObj.name
is either null
or undefined
.
The myObj.name
expression contains some value, and it’s not null
or undefined
. Therefore, the myObj.name
value will be stored inside the val
variable, and after you print the val
variable, the output you get is as follows.
Output:
The nullish
coalescing operator ??
can be used inside a single statement with any number expression like in the example below.
Code:
let val = myObj.name ?? myObj.email ?? 'something null or undefined';
console.log(val)
In this example, if both myobj.name
and myObj.email
are either null
or undefined
, then only the ??
operator will return the string, or it will return the value of any one of the first expression which is not null
or undefined
.
So, here, only the myObj.email
expression is undefined
and not myObj.name
, therefore, the value of the expression myObj.name
will be returned and stored inside the val
variable.
The val
variable will now contain the object which the name
property was storing, and this object will be printed on the console.
Output:
The operator ??
is not supported on IE and Opera browsers. For more details related to browser compatibility, you can read the MDN documentation.
Before the ??
operator was introduced inside the JavaScript programming language, a common approach was using the logical OR
operator (||)
to assign a variable’s default value. But this approach of assigning a default value creates various issues.
Code:
let val_1 = undefined || 'Hello!';
let val_2 = false || 'Hello!';
let val_3 = '' || 'Hello!';
console.log(val_1, val_2, val_3);
Output:
Hello! Hello! Hello!
The ||
being a Boolean logical operator, this operator may cause unexpected behavior if you consider 0
, ''
, or NaN
as valid values as shown above.
Changing the same example by replacing the OR
operator with the nullish
coalescing operator ??
will yield different results.
Code:
let val_1 = undefined ?? 'Hello!';
let val_2 = false ?? 'Hello!';
let val_3 = '' ?? 'Hello!';
console.log(val_1, val_2, val_3);
Output:
Hello! false
As you can see, this operator produces proper output. This is why the nullish
coalescing operator ??
was introduced in JavaScript.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn