Dynamic Object Key in JavaScript
- Understanding Object Key Access in JavaScript
- Initializing Dynamic Object Keys
- Accessing Dynamic Object Keys
- Conclusion
- FAQ
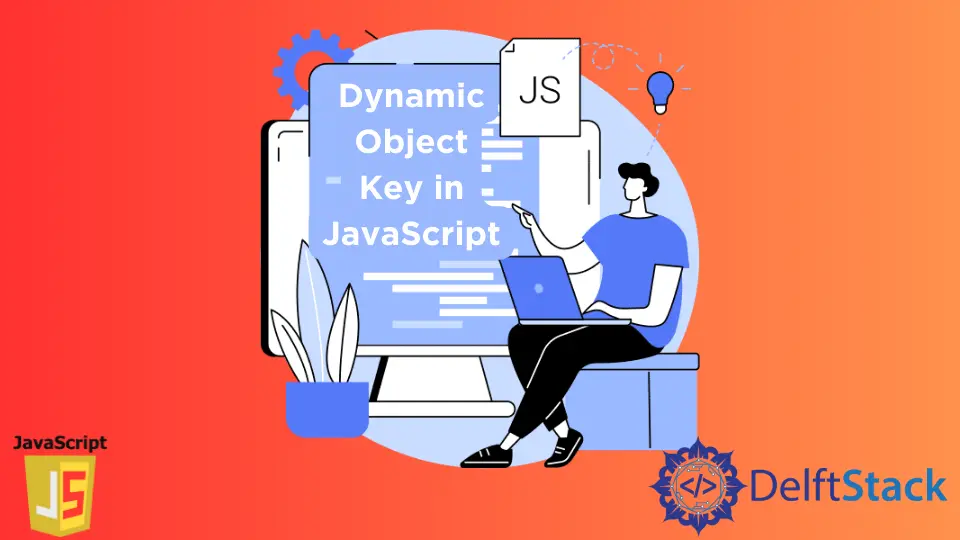
Dynamic object keys in JavaScript offer a powerful way to manage data structures. Unlike static property access, which requires predefined keys, dynamic keys allow you to set and retrieve properties using variables. This flexibility is particularly useful in scenarios where the property names are not known until runtime.
In this article, we will explore how to dynamically create object keys and access them using JavaScript’s square bracket notation. We’ll delve into practical examples and explain the concepts in a user-friendly manner, making it easy for both beginners and seasoned developers to grasp these essential techniques.
Understanding Object Key Access in JavaScript
JavaScript provides two primary methods for accessing object properties: dot notation and bracket notation. Dot notation is straightforward but limited to predefined keys. For example, if you have an object representing a person, you can access the name property like this:
const person = { name: "John", age: 30 };
console.log(person.name);
However, if you need to access a property dynamically—perhaps based on user input or other runtime data—bracket notation is the way to go. This method allows you to use variables as keys, making your code more adaptable.
Initializing Dynamic Object Keys
To initialize object keys dynamically, you can use the square bracket notation. This allows you to set properties based on variable values. Here’s a simple example:
const dynamicKey = "favoriteColor";
const person = {};
person[dynamicKey] = "blue";
console.log(person);
Output:
{ favoriteColor: 'blue' }
In this example, we create an empty object called person
. We then define a variable dynamicKey
that holds the string “favoriteColor”. Using bracket notation, we assign the value “blue” to this key in the person
object. As a result, we dynamically create a property without needing to know the key beforehand.
This approach is especially beneficial when working with data structures where keys may vary, such as when processing user inputs or API responses. By leveraging dynamic keys, you can build more flexible and robust applications.
Accessing Dynamic Object Keys
Once you’ve initialized dynamic keys, accessing their values is just as simple. You can use the same bracket notation to retrieve the values stored in those keys. Here’s how:
const dynamicKey = "favoriteColor";
const person = { favoriteColor: "blue" };
console.log(person[dynamicKey]);
Output:
blue
In this code snippet, we again use the variable dynamicKey
to access the favoriteColor
property of the person
object. The bracket notation allows us to retrieve the value associated with the dynamically created key. This method is particularly useful when dealing with a large number of properties or when the exact property names are not known until runtime.
Dynamic keys can also be used in loops or functions, making them incredibly versatile. For instance, if you have an array of keys and want to populate an object based on those keys, you can easily do so using bracket notation. This flexibility can significantly reduce the amount of hardcoding required in your applications.
Conclusion
Dynamic object keys in JavaScript empower developers to create more flexible and adaptable code. By utilizing bracket notation, you can initialize and access properties without needing to know their names in advance. This technique is invaluable when working with dynamic data, such as user inputs or API responses. Understanding how to effectively use dynamic keys will enhance your JavaScript skills and enable you to build more robust applications.
FAQ
-
What is a dynamic object key in JavaScript?
A dynamic object key allows you to set and access object properties using variables, making your code more flexible. -
How do I access a dynamic key in an object?
You can access a dynamic key using bracket notation, likeobject[variable]
. -
Can I use dynamic keys in loops?
Yes, dynamic keys can be used in loops to populate or access object properties based on varying conditions. -
What is the difference between dot notation and bracket notation?
Dot notation is used for static keys, while bracket notation allows for dynamic keys, enabling more flexible property access. -
Are dynamic keys useful in real-world applications?
Absolutely! They are particularly useful in scenarios involving user inputs, API responses, and other dynamic data structures.