JavaScript Double Pipe
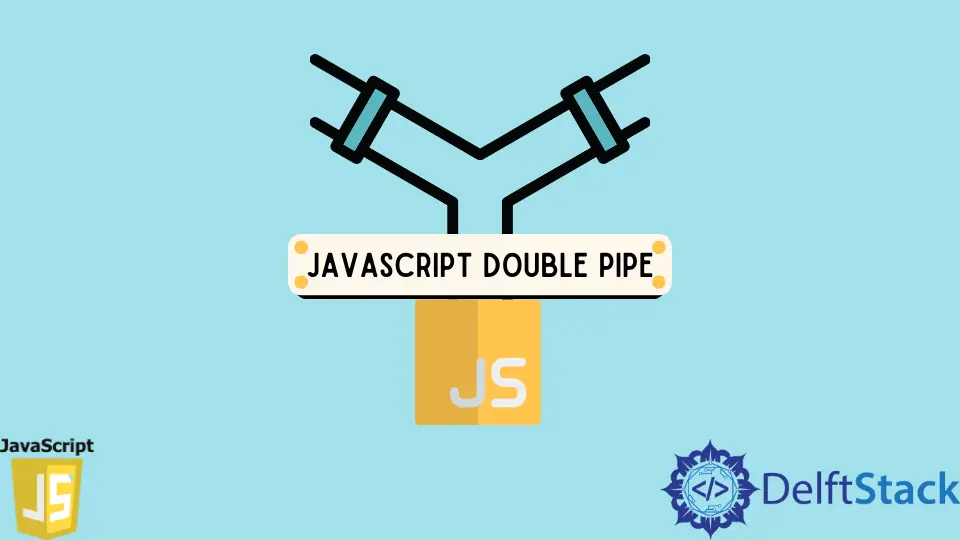
JavaScript is a versatile programming language, widely used for web development. Among its many features, the logical OR operator, represented by the double pipe symbol (||), is essential for making decisions in code. This operator evaluates two expressions and returns the first truthy value it encounters. If both values are falsy, it returns the last value. Understanding how to utilize the double pipe can enhance your coding efficiency and improve logic flow in your applications.
In this article, we will explore the JavaScript double pipe in detail, looking at its syntax, use cases, and practical examples. Whether you’re a beginner or an experienced developer, mastering this operator can significantly boost your JavaScript skills.
What is the JavaScript Double Pipe?
The JavaScript double pipe operator (||) is a logical operator that performs a short-circuit evaluation. It checks the truthiness of two expressions and returns the first one that evaluates to true. If neither expression is true, it returns the last one. This feature is particularly useful in scenarios where you want to set default values or execute conditional logic.
For instance, consider a situation where you want to assign a default value to a variable if it is undefined or null. Using the double pipe operator allows you to accomplish this succinctly.
Syntax
The syntax for using the double pipe operator is straightforward:
let result = expression1 || expression2;
Here, expression1
and expression2
are the values being evaluated. If expression1
is truthy, result
will be assigned its value; otherwise, it will take the value of expression2
.
Practical Examples of the Double Pipe Operator
Now that we’ve covered the basics, let’s dive into some practical examples to illustrate how the double pipe operator works in real-world scenarios.
Example 1: Default Value Assignment
In this example, we’ll see how the double pipe operator can be used to assign a default value to a variable.
let userInput = null;
let defaultValue = 'Default Value';
let finalValue = userInput || defaultValue;
console.log(finalValue);
Output:
Default Value
In this code snippet, the variable userInput
is set to null
, which is a falsy value. The double pipe operator checks userInput
first. Since it is falsy, finalValue
is assigned the value of defaultValue
, resulting in “Default Value” being printed to the console.
Example 2: Conditional Logic
The double pipe operator can also simplify conditional logic, making your code cleaner and more readable.
let isLoggedIn = false;
let welcomeMessage = isLoggedIn || 'Welcome, Guest!';
console.log(welcomeMessage);
Output:
Welcome, Guest!
In this example, isLoggedIn
is set to false
. The double pipe operator checks isLoggedIn
first, and since it is falsy, welcomeMessage
is assigned “Welcome, Guest!”. This usage demonstrates how the double pipe operator can streamline your conditional statements.
Example 3: Chaining Multiple Conditions
You can also chain multiple conditions using the double pipe operator to evaluate more than two expressions.
let a = 0;
let b = null;
let c = 'Hello, World!';
let message = a || b || c;
console.log(message);
Output:
Hello, World!
In this case, a
and b
are both falsy values, so the double pipe operator continues checking until it finds c
, which is truthy. Thus, message
is assigned “Hello, World!”, showcasing the operator’s ability to evaluate multiple expressions in sequence.
Conclusion
The JavaScript double pipe operator (||) is a powerful tool for developers, allowing for efficient decision-making and default value assignment. Its ability to evaluate multiple expressions and return the first truthy value makes it invaluable in various coding scenarios. By understanding how to effectively use the double pipe operator, you can write cleaner, more concise code that enhances your overall programming efficiency. Whether you’re setting default values or simplifying conditional logic, the double pipe operator is a must-have in your JavaScript toolkit.
FAQ
-
What does the double pipe operator do in JavaScript?
The double pipe operator (||) evaluates two expressions and returns the first truthy value it encounters, or the last value if both are falsy. -
Can I use the double pipe operator with non-boolean values?
Yes, the double pipe operator can be used with any data type in JavaScript, not just boolean values. -
How does short-circuit evaluation work with the double pipe operator?
Short-circuit evaluation means that the second expression is only evaluated if the first expression is falsy. If the first is truthy, the second is ignored. -
Is the double pipe operator the same as the single pipe operator?
No, the single pipe operator (|) is a bitwise OR operator, whereas the double pipe operator (||) is a logical OR operator. -
Can I chain multiple double pipe operators together?
Yes, you can chain multiple double pipe operators to evaluate more than two expressions in sequence.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn