Double Exclamation Operator Example in JavaScript
- What is the Double Exclamation Operator?
- Practical Applications of the Double Exclamation Operator
- Conclusion
- FAQ
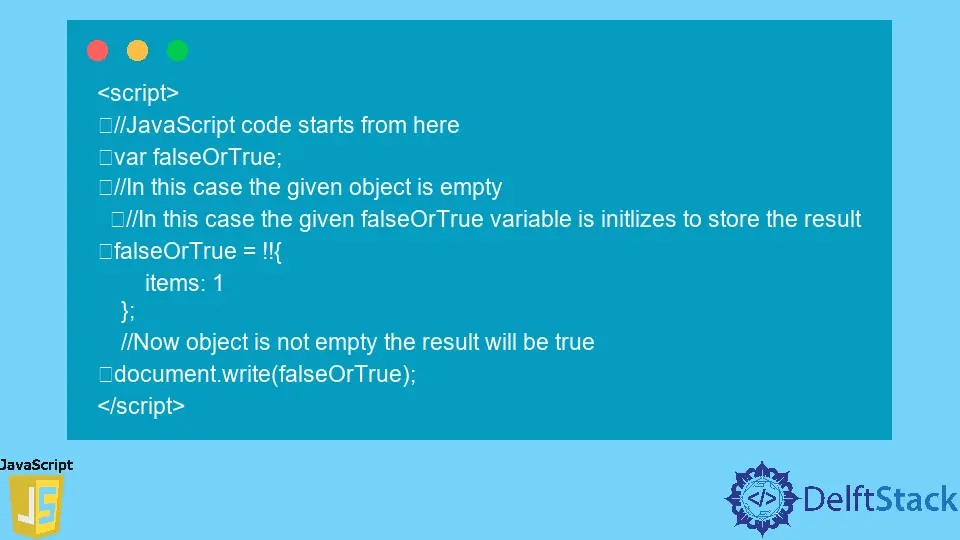
JavaScript is a versatile programming language, and one of its interesting features is the double exclamation operator, commonly known as the “not not” operator. This operator is a concise way to convert a value to a Boolean, effectively returning true
or false
. Understanding how to use the double exclamation operator can greatly enhance your ability to handle truthy and falsy values in your JavaScript code.
In this article, we will explore the double exclamation operator, provide clear examples, and explain how it works in various scenarios. Whether you are a beginner or an experienced developer, grasping this concept will undoubtedly improve your coding skills.
What is the Double Exclamation Operator?
The double exclamation operator (!!) is a simple yet powerful tool in JavaScript. It consists of two unary logical NOT operators applied in succession. The first NOT operator negates the value, and the second one negates it again, effectively converting the value into its Boolean equivalent. This operator is particularly useful when you want to ensure that a variable is treated as a Boolean value.
For instance, if you have a variable that could hold various types of values (like strings, numbers, or objects), using !! will allow you to determine its truthiness or falsiness. The double exclamation operator is a clean and efficient way to perform this conversion without needing lengthy conditional statements.
Example of Using the Double Exclamation Operator
Let’s look at a few examples to illustrate how the double exclamation operator works in practice.
let value1 = "Hello World";
let value2 = 0;
let value3 = null;
console.log(!!value1);
console.log(!!value2);
console.log(!!value3);
Output:
true
false
false
In this example, value1
is a non-empty string, which is considered truthy, so !!value1
returns true
. Conversely, value2
is 0
, a falsy value, so !!value2
returns false
. Lastly, value3
is null
, another falsy value, leading to !!value3
also returning false
. This illustrates how the double exclamation operator can effectively convert different types of values into Boolean values.
Practical Applications of the Double Exclamation Operator
The double exclamation operator can be applied in various scenarios to simplify code and improve readability. Below are some practical applications.
1. Validating User Input
When validating user input, you often need to check if a value exists or is valid. The double exclamation operator can streamline this process.
let userInput = "Sample input";
if (!!userInput) {
console.log("Input is valid");
} else {
console.log("Input is invalid");
}
Output:
Input is valid
In this case, the user input is a non-empty string, so !!userInput
evaluates to true
, and the message “Input is valid” is logged. If the input were an empty string or a falsy value, the else block would execute instead.
2. Ternary Operator Simplification
The double exclamation operator can also be used in conjunction with the ternary operator to return Boolean values concisely.
let isActive = null;
let status = !!isActive ? "Active" : "Inactive";
console.log(status);
Output:
Inactive
Here, isActive
is null
, which is falsy. Therefore, !!isActive
evaluates to false
, and the ternary operator returns “Inactive”. This method is cleaner and more readable than using a traditional if-else statement.
3. Filtering Arrays
Another practical application of the double exclamation operator is filtering arrays for truthy values. You can use it in combination with the filter
method.
let mixedArray = [0, 1, "", "text", null, undefined, 5];
let truthyValues = mixedArray.filter(Boolean);
console.log(truthyValues);
Output:
[ 1, 'text', 5 ]
In this example, we use filter(Boolean)
to retain only the truthy values from the mixedArray
. The double exclamation operator is implicitly used in the Boolean
function, which converts each element to its Boolean equivalent. The resulting array contains only the values that evaluate to true
.
Conclusion
The double exclamation operator in JavaScript is a simple yet effective tool for converting values to their Boolean equivalents. By understanding how to use this operator, you can write cleaner, more efficient code that handles truthy and falsy values with ease. Whether you’re validating user input, simplifying conditional statements, or filtering arrays, the double exclamation operator can enhance your JavaScript programming skills. So, the next time you’re working with various data types in JavaScript, remember the power of the double exclamation operator!
FAQ
-
What does the double exclamation operator do in JavaScript?
The double exclamation operator converts a value to its Boolean equivalent, returningtrue
orfalse
. -
Can the double exclamation operator be used with any data type?
Yes, it can be used with strings, numbers, objects, and other data types to determine their truthiness or falsiness. -
Is the double exclamation operator the same as using the Boolean function?
Yes, both achieve the same result, but the double exclamation operator is often more concise. -
When should I use the double exclamation operator?
Use it when you need to convert values to Boolean types, especially in conditional statements or validations. -
Are there any performance implications of using the double exclamation operator?
Generally, there are no significant performance concerns; it’s a lightweight operation in JavaScript.