How to Enable/Disable Input Button Using JavaScript
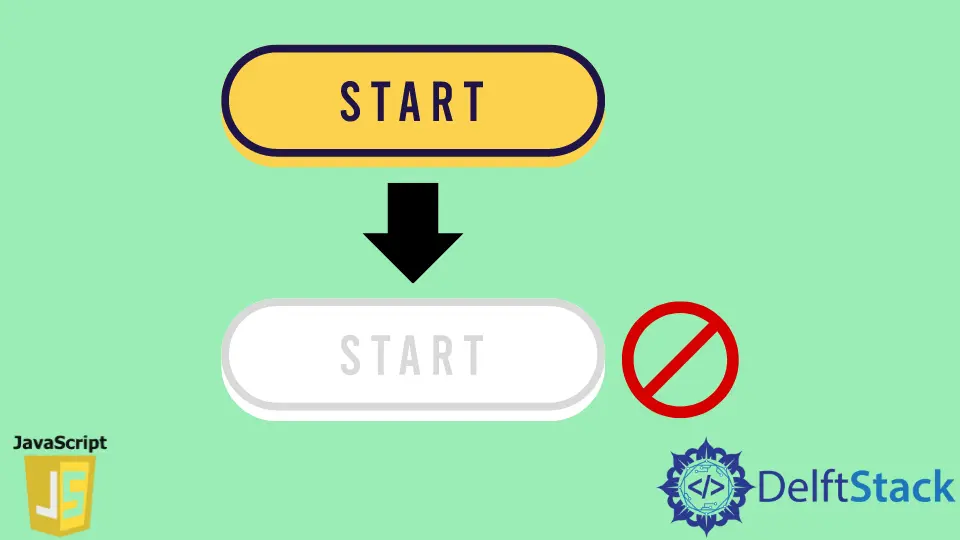
This tutorial teaches how to enable/disable an HTML input button using JavaScript/JQuery.
We often come across situations where we want to enable/disable an HTML button, like before and after submitting a form. The best way to do this is to use the JavaScript DOM Manipulation to select the button and change its disabled
status to true
/false
to toggle its on
/off
state. Below, we will see two ways to disable/enable a button, one using JavaScript and the other using JQuery.
Use JavaScript to Enable/Disable Input Button
Below is the demonstration of a button getting activated when text is input in a box and deactivated otherwise.
HTML Code
<input class="input" type="text" placeholder="fill me">
<button class="button">Click Me</button>
JavaScript Code
let input = document.querySelector('.input');
let button = document.querySelector('.button');
button.disabled = true;
input.addEventListener('change', stateHandle);
function stateHandle() {
if (document.querySelector('.input').value === '') {
button.disabled = true;
} else {
button.disabled = false;
}
}
Here, we first store the reference to input and button in two variables and then set the button’s default state to disabled. We add an event listener to see if there is any input activity inside the textbox and then use the function stateHandle()
to disable/enable the submit button accordingly.
Use jQuery to Enable/Disable Input Button
<html>
<head>
<title>Enable/Disable a HTML button</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
</head>
<body>
Name: <input type="text" id="reco" />
<input type="submit" id="submit" disabled="disabled" />
</body>
<script>
$(document).ready(function () {
$('#reco').on('input change', function () {
if ($(this).val() != '') {
$('#submit').prop('disabled', false);
}
else {
$('#submit').prop('disabled', true);
}
});
});
</script>
</html>
We use the ready()
function to make it available once the document has been loaded. The .on()
method then attaches the event handler to the input field. The change
event will check for changes in the input field and run the function accordingly. The .prop()
method then changes the state of the button.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn