How to Convert Decimal to Hexadecimal Number in JavaScript
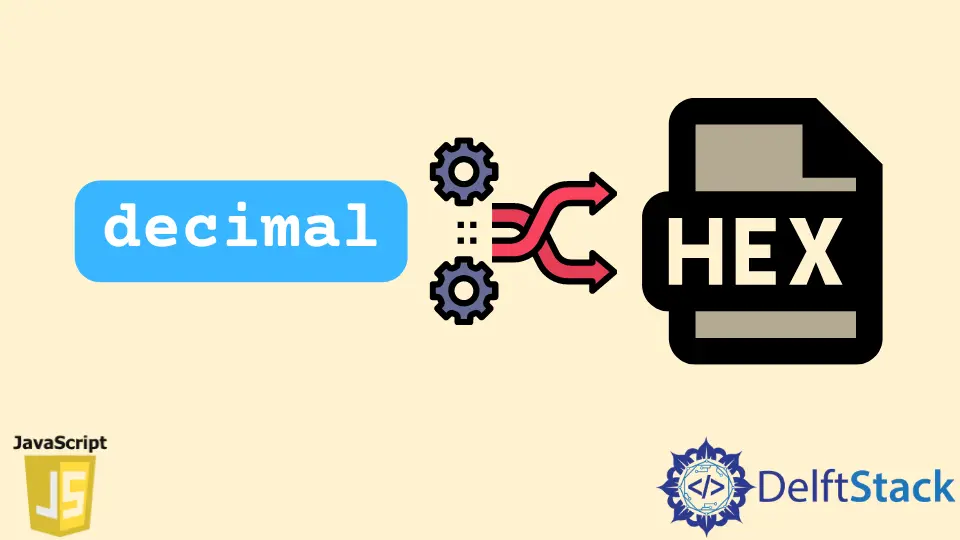
Back in the day, humans have created various types of number systems. Initially, there was a unary number system where we used stones to do the counting. Later we discovered other number systems like decimal, octal, hexadecimal, and so on. When it comes to programming, the most popular number system is the binary number system.
In programming, we always try to do number conversion from one form to another form. And because of built-in functions provided by various modern programming languages like JavaScript, our task of number conversion becomes much easier. This article will show how to convert any decimal number into its equivalent hexadecimal number using the toString()
JavaScript function.
Convert Decimal to Hexadecimal Number Using toString()
Function in JavaScript
To convert decimal numbers to hexadecimal numbers, you can use the toString()
function. The toString()
is a pre-defined function in python. This function takes a parameter called radix, which is a decimal number. The value of the radix can range from 2 to 36 (both including).
You have to pass the base of the number to which you want to convert the given number to. For example, In this case, we will pass 16
to the toString()
function because we want to convert our decimal number to hexadecimal, and the base of the hexadecimal number is 16
.
function decToHex(num) {
return num.toString(16)
}
console.log(decToHex(15));
Output:
f
We have created a function called dexToHex()
, which takes decimal number num
as an input. Then with the help of the toString()
function, we will convert that decimal number into a hexadecimal number by passing the base 16 into it. Lastly, the function will return the hexadecimal number, and it will be printed in the console.
Since we passed the decimal number 15 as the input to the decToHex()
function, the hex number returned will be f
because number 15 in hexadecimal is f
. You can also change the output to uppercase F
using the toUpperCase()
function as follows.
function decToHex(num) {
return num.toString(16).toUpperCase()
}
console.log(decToHex(15));
Output:
F
To learn more, you can check the decimal to hexadecimal conversion table here.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn