How to Make A Custom sort() Function in JavaScript
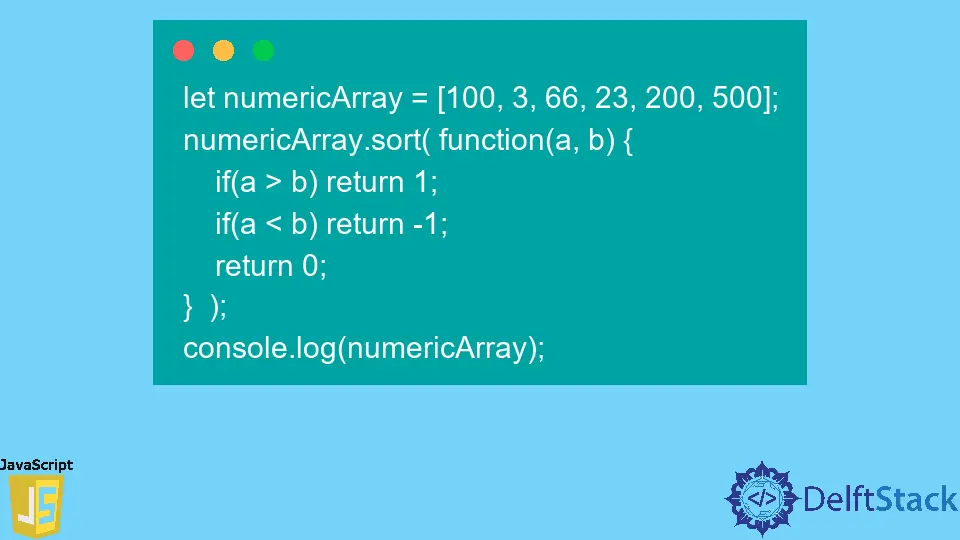
In this article, we’ll be discussing the sort operation over JavaScript arrays.
the sort()
Method in JavaScript
The sort()
method is used to order the elements in an array. By default, this method sorts elements in ascending order.
Usually, this method modifies the original array by changing the positions of elements. In addition, the sort()
method casts input array elements to strings and then does a string comparison to sort elements.
If you input an array of numbers, those numbers will be first converted to their string value and then compared. Let’s create an array of numbers and use the sort()
method to sort it.
Code Example:
let numericArray = [100, 3, 66, 23, 200, 500];
numericArray.sort();
console.log(numericArray);
Output:
This is not ordered as expected. We have to customize the sort()
method to fix this.
It allows us to pass a custom compare function as an argument. This compare function accepts two arguments, and the applied rules on the passed arguments will determine the sort order.
Use Custom sort()
Method to Sort Array of Numbers in JavaScript
To sort an array of numbers, we should implement a compare function as shown in the following.
if the returned values from compare(a, b)
function is less than 0, the sort()
method will position a before b
.
In the opposite case, b
will be positioned before a
. Whenever, the compare(a, b)
function returns 0
, the positions of a
and b
will not change.
Hence, the custom compare function can be passed to the sort()
method, as shown in the following.
Code Example:
let numericArray = [100, 3, 66, 23, 200, 500];
numericArray.sort(function(a, b) {
if (a > b) return 1;
if (a < b) return -1;
return 0;
});
console.log(numericArray);
Output:
As expected, the array has been sorted properly now. This is a very useful feature of the JavaScript sort()
method that users can decide the ordering.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.