How to Set Current Year Copyright in JavaScript
-
Use the
getFullYear()
Method for Current Year of Copyright in JavaScript -
Use the
Regex
andexec()
Method for Current Year of Copyright in JavaScript
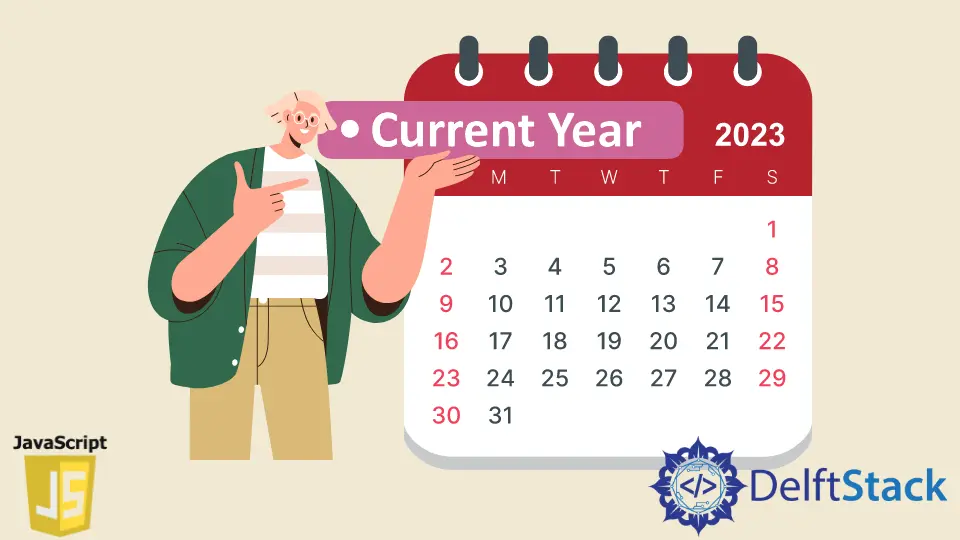
In the development phase of a web app or similar tech product, it is not wise to manually set the copyright year every time.
Using JavaScript, it is easier to set a function or method to automatically change the date whenever it is updated.
Use the getFullYear()
Method for Current Year of Copyright in JavaScript
Generally, the new Date()
object returns the date according to the browser’s time zone. And the return format is a string
.
The getFullYear()
method simplifies the way of a reinitialization of years in every modification or change. This procedure makes a product more dynamic and reliable.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
</head>
<body>
<script>document.write(new Date().getFullYear())</script>
</body>
</html>
Output:
Use the Regex
and exec()
Method for Current Year of Copyright in JavaScript
Whenever the Date()
returns the value, it is in the string form and the initial start with the year.
We will generate a regex
that will execute the first literals of the year. Here, we will use a regex
to grab 4 characters and not calculate years after 9999
.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
</head>
<body>
<script>document.write(/\d{4}/.exec(Date())[0])</script>
</body>
</html>
Output:
Related Article - JavaScript Date
- How to Add Months to a Date in JavaScript
- How to Add Minutes to Date in JavaScript
- How to Add Hours to Date Object in JavaScript
- How to Calculate Date Difference in JavaScript
- How to Calculate Age Given the Birth Date in YYYY-MM-DD Format in JavaScript
- How to Get First and Last Day of Month Using JavaScript