How to Convert JSON Object Into String in JavaScript
- Create a JSON Object in JavaScript
-
Convert a JSON Object Into a String Using
JSON.stringify()
in JavaScript
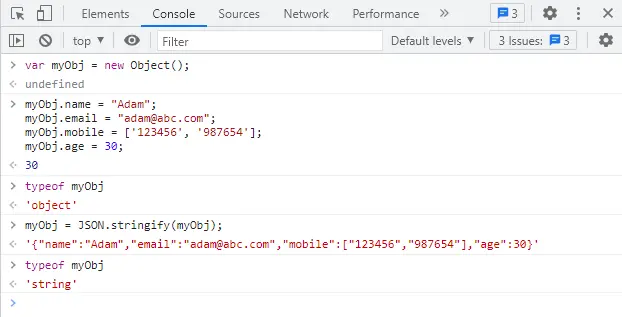
This article will discuss and demonstrate how to create a JSON object and convert it into a string to send it back to a server.
Create a JSON Object in JavaScript
First, we must create an object of the Object
class using the new
keyword. Then, we can store its reference inside the variable, as shown below.
var myObj = new Object();
To add properties inside the object, we can use the dot notation. In myObj
, we add four properties: name, email, mobile (an array), and age.
myObj.name = 'Adam';
myObj.email = 'adam@abc.com';
myObj.mobile = ['123456', '987654'];
myObj.age = 30;
The good thing about the object is that we can assign any data type to a property of the object. In the example above, we see that we have assigned a string, array, and number as the values to the object’s properties.
If we print the data type of the myObj
using the type
keyword, we will get the type as an object.
typeof myObj
Output:
'object'
If we want to send this object to the server, it must be in string format. That is why we first need to convert this object into a string before sending it.
Convert a JSON Object Into a String Using JSON.stringify()
in JavaScript
The JSON.stringify()
method is used to parse the JavaScript object into a JSON string.
Syntax:
JSON.stringify(value)
JSON.stringify(value, replacer)
JSON.stringify(value, replacer, space)
The value
(mandatory) can be an array or an object. The replacer
(optional) alters the stringification
process.
The space
(optional) parameter takes an integer value and inserts space (including indentation, line break characters, etc.). This parameter can be used for readability purposes.
If the space
parameter is not specified, no space will be added in the output JSON string. The default value for the replacer
function and the space
parameter is null
.
To convert the JavaScript object myObj
which we have created, we will directly pass it as a parameter to the JSON.stringify()
method as shown below.
myObj = JSON.stringify(myObj);
After converting the JavaScript object, we will store it inside myObj
. Finally, if we check the data type of myObj
, we’ll see string
instead of object
, as shown below.
typeof myObj
Output:
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn