How to Create and Save File in JavaScript
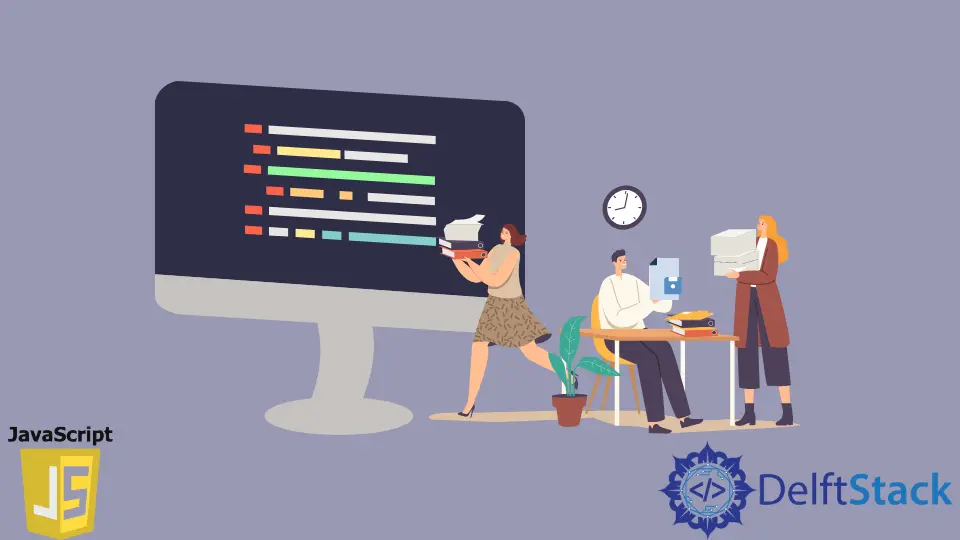
This tutorial teaches how to create and save a file in JavaScript.
It is easy to create files and store them on the server side NodeJS, but there are few options to do so on the client side. In this tutorial, we write a custom function that helps us create files using data and then save it. In modern browsers, we get a function called msSaveOrOpenBlob that helps us to save a file. But in older browsers, we first generate a link to the file and then download it by adding the download
attribute on the anchor tag.
function downloadFiles(data, file_name, file_type) {
var file = new Blob([data], {type: file_type});
if (window.navigator.msSaveOrOpenBlob)
window.navigator.msSaveOrOpenBlob(file, file_name);
else {
var a = document.createElement('a'), url = URL.createObjectURL(file);
a.href = url;
a.download = file_name;
document.body.appendChild(a);
a.click();
setTimeout(function() {
document.body.removeChild(a);
window.URL.revokeObjectURL(url);
}, 0);
}
}
In the above function, we check if the browser has support for msSaveOrOpenBlob
, and if found, we use it to save the file. Otherwise, we create an anchor tag pointing to the created file. We add the download attribute to the anchor tag and attach that tag to the document’s body. We use JavaScript to click on it, which triggers the download, and in this way, we save the file. We then remove that anchor tag from the body and revoke the created URL.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn