How to Convert Character Code to ASCII Code in JavaScript
-
Use the
String.charCodeAt()
Function to Convert Character to ASCII in JavaScript -
Use the
String.codePointAt()
Function to Convert Character to ASCII in JavaScript
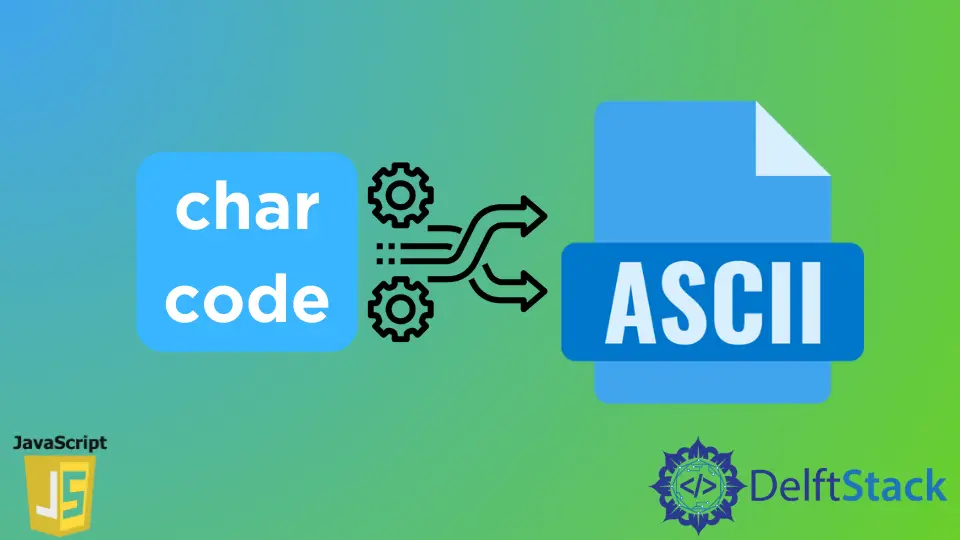
This tutorial teaches how to convert character code to ASCII(American Standard Code for Information Interchange
) code. ASCII code is just a numeric value assigned to characters and symbols. It is useful in the storage and manipulation of characters.
Use the String.charCodeAt()
Function to Convert Character to ASCII in JavaScript
The charCodeAt()
function defined on string prototype returns the Unicode value i.e. UTF-16
code at the specified index. It returns a value in the range 0
to 216 - 1 i.e. 65535
. The codes 0
to 127
in UTF codes are same as the ASCII code. So, we can use the charCodeAt()
function to convert character codes to ASCII codes.
var x = 'B';
var ascii_code = x.charCodeAt(0);
console.log(ascii_code);
Output:
66
We can return the original character by using the fromCharCode()
function.
Use the String.codePointAt()
Function to Convert Character to ASCII in JavaScript
The codePointAt()
method defined on the string prototype returns the code point value of the character. Like charCodeAt
, it also requires the index of the character to return the codepoint value of the character from the string, but unlike charCodeAt
does not return the UTF-16
code unit and hence can handle codepoints beyond the ASCII code 127
.
var x = 'B';
var ascii_code = x.codePointAt(0);
console.log(ascii_code);
Output:
66
We can return the original character by using the fromCodePoint()
function.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn