JavaScript Ternary Conditional Operator
- Example: JavaScript Ternary Conditional Operator
- Example: JavaScript Nested Ternary Operators
- Example: Multiple Operations in the JavaScript Ternary Operator
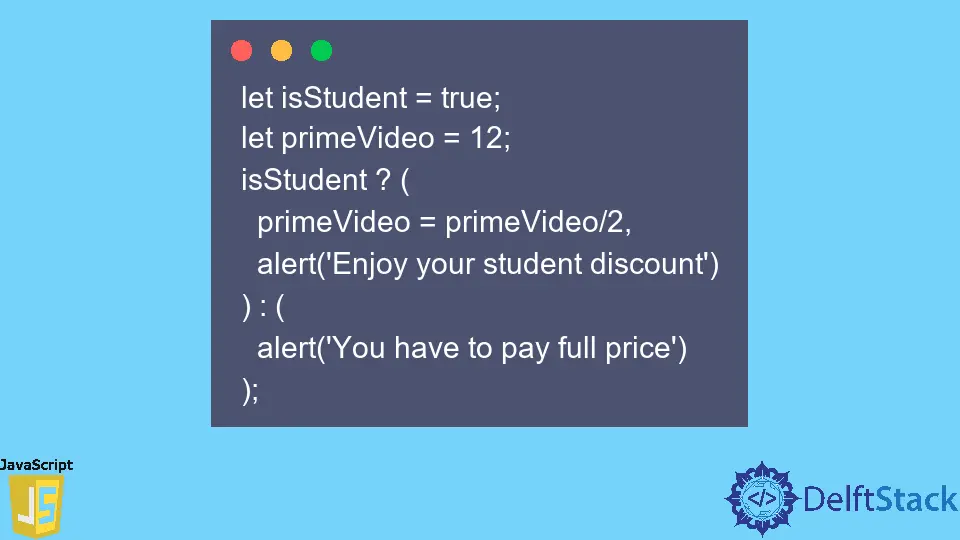
This tutorial will introduce how to use the ?:
conditional operator in JavaScript.
The if ... else
statement helps us execute a certain code block only if a certain condition is met. The conditional operator, also known as the ternary operator, is a one-line shorthand for if ... else
statements. It helps to write clean and concise code. It is the only JavaScript operator that requires 3 operands: The condition to evaluate, an expression to execute if the condition is true
, and an expression to execute if the condition is false
. Since it takes 3 operands, its name is the ternary operator.
condition ? expression1 : expression2
The ternary operator first evaluates the given condition
. The condition is separated from expression1
by a ?
and expression2
is separated from expression1
by a :
. If the condition
is true, then the conditional operator executes the expression1
, else it executes expression2
.
Example: JavaScript Ternary Conditional Operator
var age = 18;
var canVote;
if (age >= 18) {
canVote = 'yes';
} else {
canVote = 'no';
}
The above example shows a conditional statement executed using the traditional if ... else
statement.
var age = 18;
var canVote = age >= 18 ? 'yes' : 'no';
We have rewritten the above piece of code using the ternary operator.
Example: JavaScript Nested Ternary Operators
Like the if ... else
statement, we can also use nested ternary operators to perform multiple condition checking.
var carSpeed = 90;
var warning =
speed >= 100 ? 'Way Too Fast!!' : (speed >= 80 ? 'Fast!!' : 'Nice :)');
console.log(warning);
In the above code, we generate a warning for the car based on car speed. First, we check if carSpeed
is over 100 and if the condition is satisfied, we generate a warning saying the car is moving Way Too Fast!!
. Otherwise, we have nested a second expression checking whether the carSpeed
is greater than 80 and display Fast
/ Nice
depending on the evaluation.
Example: Multiple Operations in the JavaScript Ternary Operator
We can run multiple operations inside a ternary operator just like if ... else
statements.
let isStudent = true;
let primeVideo = 12;
isStudent ?
(primeVideo = primeVideo / 2, alert('Enjoy your student discount')) :
(alert('You have to pay full price'));
In the above code, we perform two operations instead of one, changing the value of primeVideo
to its half and alert the user.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn