How to Detect Clipboard Data in JavaScript
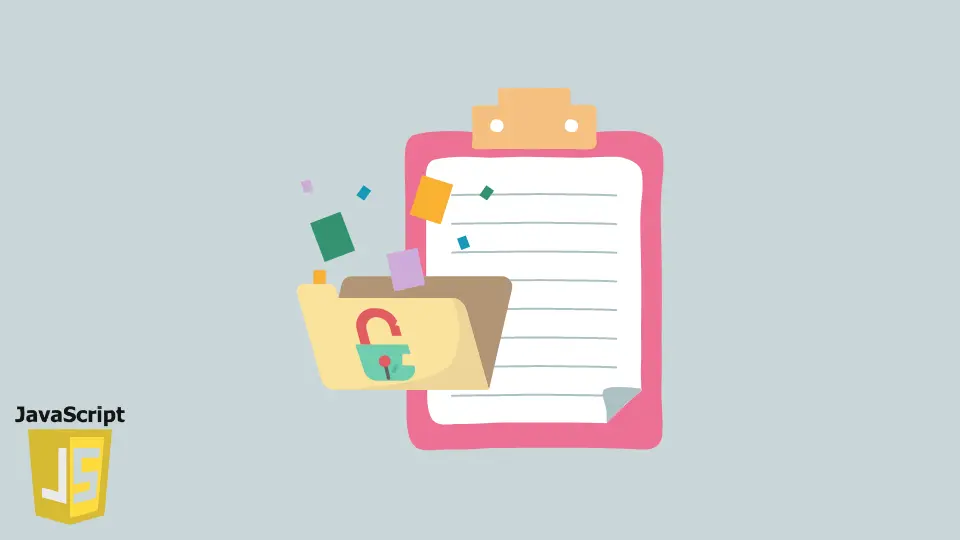
Today’s post will teach detecting clipboard data on paste
events in JavaScript.
JavaScript Clipboard Data
The paste
event is raised when the user initiates a paste
action through the browser UI.
When the cursor is in an editable context, the default action is to paste the clipboard’s contents into the document at the cursor position. A handler for this event can access the clipboard’s contents by calling getData()
on the event’s clipboardData
property.
An event handler must cancel the default action with the event.preventDefault()
and manually insert the desired data to override the default behavior. It is possible to create and send a synthetic insert event, but this does not affect the document’s content.
The EventTarget
interface’s addEventListener()
method configures a function to be called whenever the specified event is delivered to the target.
Let’s take an example, define two div elements. One with the source and one with the destination.
Source Div will contain the data to be copied from, and the destination will be where to paste the copied data.
<div class="source">Hello World!</div>
<div class="destination" contenteditable="true">...Good Bye!</div>
const destinationElement = document.querySelector('div.destination');
destinationElement.addEventListener('paste', (event) => {
const paste = (event.clipboardData || window.clipboardData).getData('text');
console.log(paste);
const selection = window.getSelection();
selection.deleteFromDocument();
selection.getRangeAt(0).insertNode(document.createTextNode(paste));
event.preventDefault();
});
In the above example, we first select the destination element using the querySelector
. Once the element is selected, we listen for the paste
event.
As soon as the user pastes the data, clipboard data is extracted from the event.
Original content is deleted from the destination element, and new content is inserted into the destination element. Try to run the above code in any browser that supports the paste
event; you will get the below result.
Before:
After:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn