How to Clear Textbox in JavaScript
- Method 1: Clearing a Textbox Using a JavaScript Function
- Method 2: Clearing a Textbox Using an HTML Attribute
- Conclusion
- FAQ
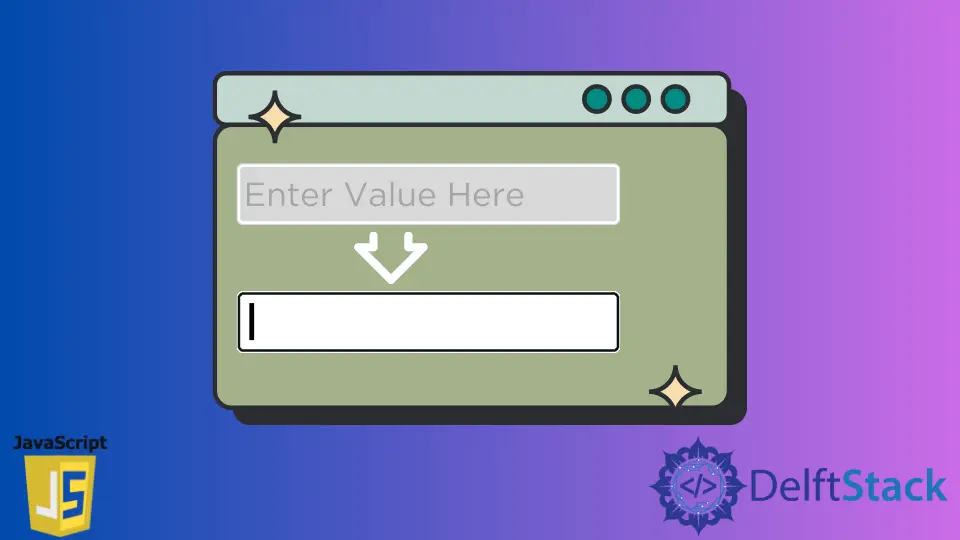
Clearing a textbox in JavaScript is a fundamental task that many developers encounter. Whether you’re building a simple form or a more complex web application, knowing how to effectively clear textboxes can enhance user experience.
In this tutorial, we will explore two methods for clearing a textbox: using a JavaScript function and leveraging an HTML attribute to trigger that function. By the end, you’ll have a clear understanding of how to implement these techniques in your projects. Let’s dive in and make those textboxes clear!
Method 1: Clearing a Textbox Using a JavaScript Function
The first method we will discuss involves creating a JavaScript function that clears the contents of a textbox. This method is straightforward and allows for more control over the clearing process.
Here’s how you can do it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Clear Textbox Example</title>
<script>
function clearTextbox() {
document.getElementById("myTextbox").value = "";
}
</script>
</head>
<body>
<input type="text" id="myTextbox" value="Type something here...">
<button onclick="clearTextbox()">Clear Textbox</button>
</body>
</html>
Output:
The textbox will be cleared when you click the button.
In this example, we have an HTML input element with an ID of myTextbox
. The JavaScript function clearTextbox
sets the value of the textbox to an empty string when called. By clicking the button, the user triggers this function, effectively clearing the textbox. This method is efficient and easy to implement, making it a popular choice for many developers.
Method 2: Clearing a Textbox Using an HTML Attribute
The second method involves using an HTML attribute to clear the textbox. This approach is even more straightforward because it combines the functionality directly into the HTML markup without needing a separate JavaScript function.
Here’s how to implement this method:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Clear Textbox Example with HTML Attribute</title>
</head>
<body>
<input type="text" id="myTextbox" value="Type something here...">
<button onclick="document.getElementById('myTextbox').value = '';">Clear Textbox</button>
</body>
</html>
Output:
The textbox will be cleared when you click the button.
In this example, we again have a textbox with an ID of myTextbox
. However, instead of defining a separate JavaScript function, we directly use the onclick
attribute of the button to clear the textbox. When the button is clicked, the value of the textbox is set to an empty string. This method is incredibly efficient for simple tasks and is often favored for its simplicity.
Conclusion
Clearing a textbox in JavaScript is a simple yet crucial task that can significantly improve the user experience of your web applications. In this tutorial, we explored two effective methods: using a JavaScript function and leveraging an HTML attribute. Each method has its advantages, allowing developers to choose based on their specific needs and project requirements. By mastering these techniques, you can enhance your web development skills and create more interactive and user-friendly applications.
FAQ
-
How do I clear multiple textboxes at once?
You can create a JavaScript function that targets all the textboxes by using a loop or selecting them with a common class name. -
Can I clear a textbox after form submission?
Yes, you can clear a textbox after submission by calling the clear function in the form’sonsubmit
event. -
Is it possible to clear a textbox using jQuery?
Absolutely! You can use jQuery’sval('')
method to clear the textbox easily. -
What happens if I try to clear a non-existent textbox?
If you try to clear a non-existent textbox, JavaScript will throw an error. Always ensure the element exists before attempting to manipulate it.
- Are there any accessibility considerations when clearing textboxes?
Yes, ensure that the action of clearing the textbox is clearly communicated to users, especially those using assistive technologies.