How to Clear Cache in JavaScript
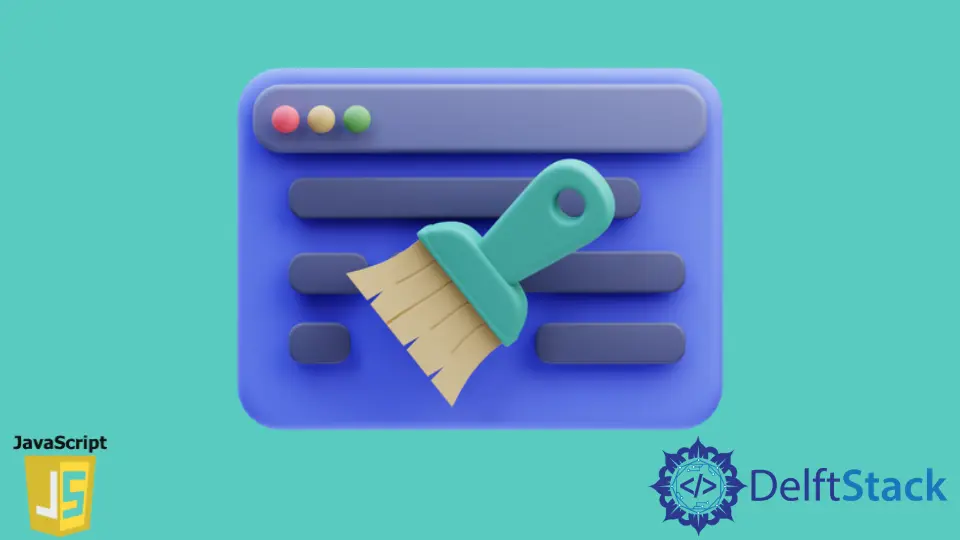
When you visit a website, your browser takes over parts of the page and stores them on your computer’s hard drive. Browsers often store so-called static assets of parts of a website that don’t change from one visit.
In today’s post, we’ll learn to clear cache in JavaScript.
Clear Cache in JavaScript
A browser is designed to save all brief caches. This is because the cache memory is the main reason for the website to load faster.
So there is no direct way to permanently clear your cache unless certain encodings in your HTML are changed. There may be a few other ways to accomplish this, but these two are the simplest and most effective.
A web browser does not allow you to clear its cache like mobile applications. While we cannot clear the entire client browser cache, loading the web page without caching using meta
tags within the HTML code is still possible.
The only way to do this is to change the code that says the browser doesn’t remember recently loaded memory, which is nothing more than cache memory.
The following two examples explain how to clear the cache. The following codes cannot be run as-is and have no output.
It must be added to the existing code to see the outputs.
Approach 1
<meta http-equiv='cache-control' content='no-cache'>
<meta http-equiv='expires' content='0'>
<meta http-equiv='pragma' content='no-cache'>
Add this part of HTML code, making the browser not record the cache memory.
Approach 2
Upload a parameter to the filename within the script
tag. Exchange it when you exchange the record.
Allow this to be the name of the document. On every occasion you load this page, you alternate the version of the script.
<script src = "oldFile.js?version = 0.1"></script>
The next time you load this page, it should look something like this.
<script src = "newfile.js?version = 0.2"></script>
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn