How to Check for an Empty String in JavaScript
-
Use the
===
Operator to Check if the String Is Empty in JavaScript -
Use the
length
Property to Check if the String Is Empty in JavaScript - Convert the Variable to Boolean to Check if the String Is Empty in JavaScript
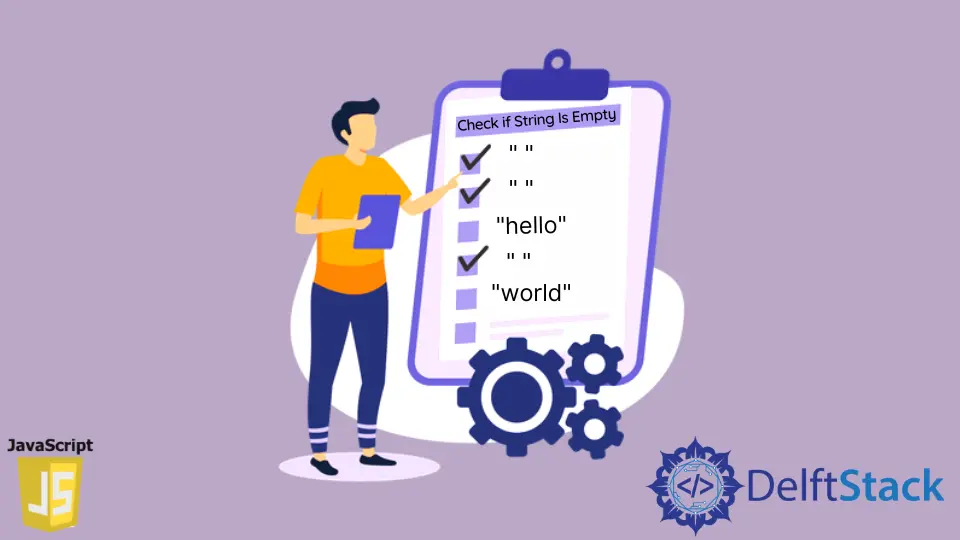
This tutorial will introduce how to check if the string is empty. Every method will have a code example, which you can run on your machine.
Use the ===
Operator to Check if the String Is Empty in JavaScript
We can use the strict equality operator (===
) to check whether a string is empty or not. The comparison data===""
will only return true
if the data type of the value is a string, and it is also empty; otherwise, return false
.
Example:
console.log(false === '')
console.log(undefined === '')
console.log(2 === '')
console.log(null === '')
console.log('Hello World!' === '')
console.log('' === '')
Output:
false
false
false
false
false
true
==
because data == ""
will return true
if data
is 0
or false
but not only the emtpy string ""
.Example:
var str1 = ''
var str2 = 0
var str3 = false
console.log(str1 == '')
console.log(str2 == '')
console.log(str3 == '')
Output:
true
true
true
Use the length
Property to Check if the String Is Empty in JavaScript
Here is another way to check JavaScript empty string. If the length is zero, then we know that the string is empty.
Example:
let str1 = 'Hello world!';
let str2 = '';
let str3 = 4;
console.log(str1.length === 0)
console.log(str2.length === 0)
console.log(str3.length === 0)
Output:
false
true
false
Convert the Variable to Boolean to Check if the String Is Empty in JavaScript
There are two ways to convert variables to a Boolean value. First by dual NOT operators (!!
), and Second by typecasting (Boolean(value))
.
Boolean(str);
!!str;
The str
is a variable. It returns false
for null
, undefined
, 0
, 000
, ""
and false
. It returns true
for not empty string and whitespace.
Example:
let str1 = 'Hello world!';
let str2 = '';
console.log(!!str1)
console.log(!!str2)
console.log(Boolean(str1))
console.log(Boolean(str2))
Output:
true
false
true
false