How to Check if a Variable Is String in JavaScript
- String Representation in JavaScript
-
Check if a Variable Is a String in JavaScript Using the
typeof
Operator
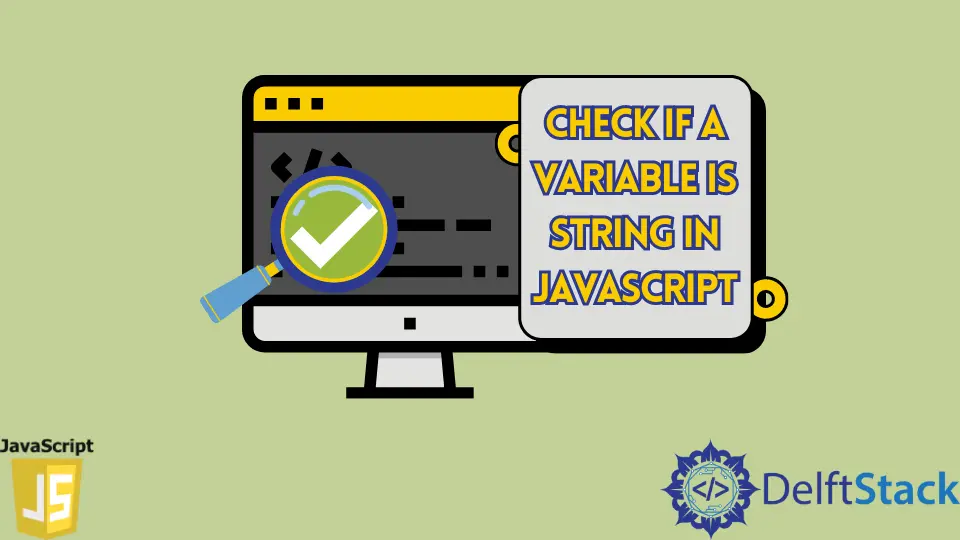
In this tutorial, we will learn how to check any variable to know whether it is a String or not.
First things first, we need to know how strings can be represented in JavaScript. Then we will learn how to check if the variable is a string or not.
String Representation in JavaScript
In JavaScript, a string is textual data stored. It can be defined in multiple ways within either single quotes, double quotes, or backticks, and also, it can be defined by using the String
class, so some strings can also be of type Objects
.
Example:
var singleQuotes = 'Hello String with Single Quotes';
var doubleQuotes = 'Hello String with Double Quotes';
var backticksText = 'Hello String with Backticks';
var stringObject = new String('Hello String Object');
console.log(singleQuotes);
console.log(doubleQuotes);
console.log(backticksText);
console.log(stringObject);
Output:
Hello String with Single Quotes
Hello String with Double Quotes
Hello String with Backticks
String {"Hello String Object"}
Check if a Variable Is a String in JavaScript Using the typeof
Operator
In JavaScript, we have the typeof
operator, which returns the type of any variable and lets us know what kind of data type we are dealing with.
If we want to know what are the types of variables used in the above example, we can write:
console.log(typeof singleQuotes);
console.log(typeof doubleQuotes);
console.log(typeof backticksText);
console.log(typeof stringObject);
Output:
string
string
string
object
After understanding how we can know the data type of a variable, checking if the variable is string can be straightforward.
If the inputText typeof
return value is string
, then that variable is a primitive String
data type. If the inputText is an instance from the String
class, it is also a string; otherwise, it can not be a string.
Let’s see the following Example:
function isString(inputText) {
if (typeof inputText === 'string' || inputText instanceof String) {
// it is string
return true;
} else {
// it is not string
return false;
}
}
var textOne = 'Hello String';
var textTwo = new String('Hello String Two');
var notString = true;
console.log('textOne variable is String > ' + isString(textOne));
console.log('textTwo variable is String > ' + isString(textTwo));
console.log('notString variable is String > ' + isString(notString));
Output:
textOne variable is String > true
textTwo variable is String > true
notString variable is String > false