How to Change Text in JavaScript
-
Change Text of an Element Using the
textContent
Property in JavaScript -
Change Text of an Element Using the
createTextNode()
Function in JavaScript
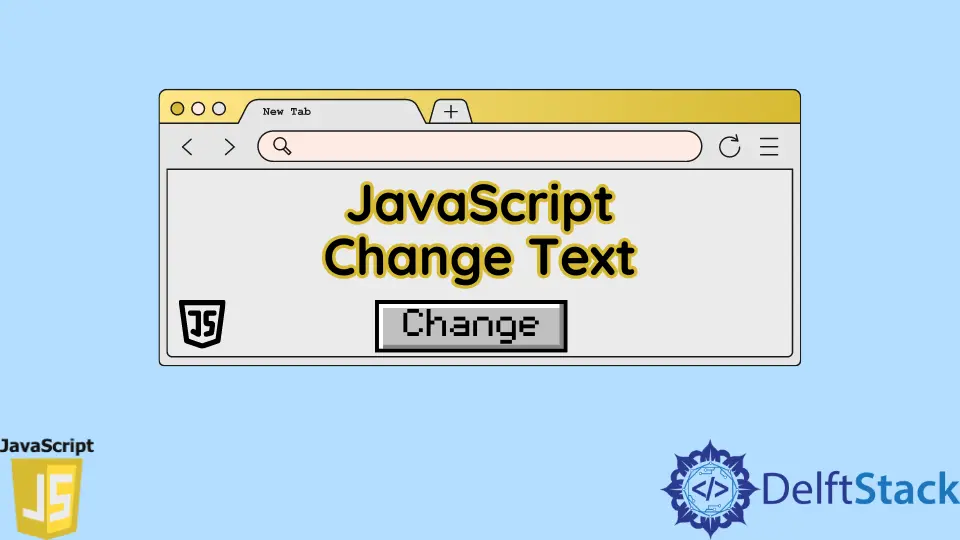
This tutorial will discuss how to change text of an element using the textContent
property and createTextNode()
function in JavaScript.
Change Text of an Element Using the textContent
Property in JavaScript
If you want to replace the old text of an element with some new text, you need to get that element using its id or class name, and then you can replace the old text with the new text using the textContent
property. If an id or class name is not specified, you can use the id
or class
attribute to give the element an id or class name. Ensure the id or class name is unique; otherwise, any element having the same id will also be changed. For example, let’s create a text element using the span
tag and change its text using the textContent
function in JavaScript. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<span id="SpanID"> Old Text </span>
<script type="text/javascript">
document.getElementById("SpanID").textContent="New Text";
</script>
</body>
</html>
Output:
New Text
The JavaScript code is placed inside the HTML file in the above code, but you can separate them if you want. You can also specify the condition on which the text should be changed like, clicking a button. If you don’t want to replace the text but append some new text with the old text, you can use the below method.
Change Text of an Element Using the createTextNode()
Function in JavaScript
If you want to append the new text with the old text, you need to get that element using its id or class name and then using the createTextNode()
function, you can create a node of the new text and using the appendChild()
function you can append the new text with the old text. If an id or class name is not specified, you can use the id
or class
attribute to give the element an id or class name. Ensure the id or class name is unique; otherwise, any element having the same id will also be changed. For example, let’s create a text element using the span
tag and append its text using the createTextNode()
function in JavaScript. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<span id="SpanID"> Old Text </span>
<script type="text/javascript">
Myspan = document.getElementById("SpanID");
Mytxt = document.createTextNode("New text");
Myspan.appendChild(Mytxt);
</script>
</body>
</html>
Output:
Old Text New Text
As you can see in the output, the new text is concatenated with the old text.