How to Change Selected Option in JavaScript
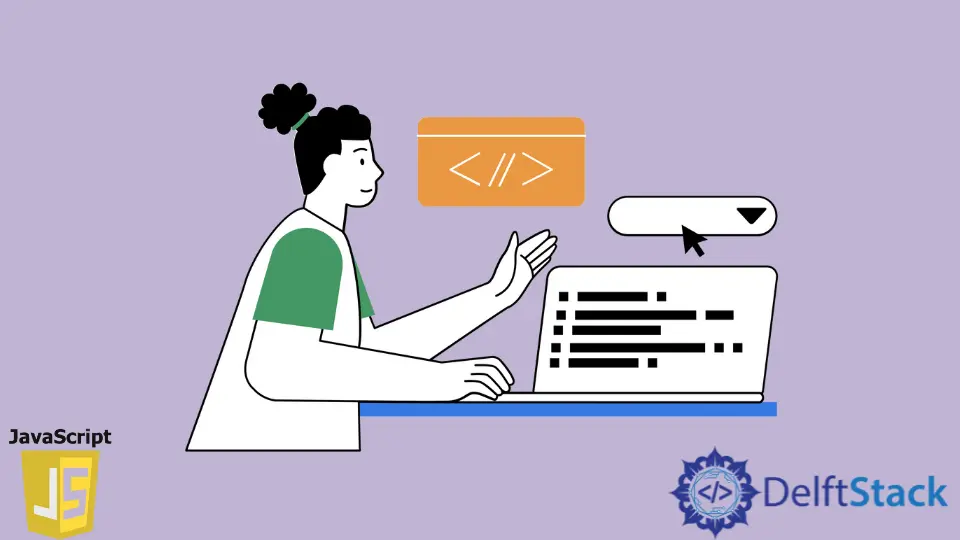
In today’s post, we’ll learn to change the selected option of the Select
element in JavaScript.
Change Selected Option in JavaScript
JavaScript provides an in-built Document
querySelector()
method that returns the primary element inside the document that fits the desired selector or set of selectors. Null
is returned if no matches are found.
Syntax:
querySelector(selectors)
selectors
are a string that is a compulsory parameter. This string contains one or more selectors that need to be matched.
This string must be a valid CSS selector string; if not, a SyntaxError
exception is thrown. For more information about selectors and how to manage them, see .querySelector()
.
This JavaScript method returns an Element
object representing the first element in the document that matches the specified set of CSS selectors or returns null
if there are no matches.
Let’s understand it with an example.
<select id="city">
<option value="mumbai" selected>Mumbai</option>
<option value="goa">Goa</option>
<option value="delhi">Delhi</option>
</select>
const selectedValue = 'goa';
document.querySelector('#city [value="' + selectedValue + '"]').selected = true;
The above example has defined the select
dropdown for selecting the preferred location. Suppose the default preferred location is Mumbai
.
Through the querySelector()
method, we can set the selected value to true
and select the default location to be goa
. The selected attribute accepts a Boolean value.
Run the above code snippet in any browser that supports JavaScript, showing the below result.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn