How to Change innerHTML Using JavaScript
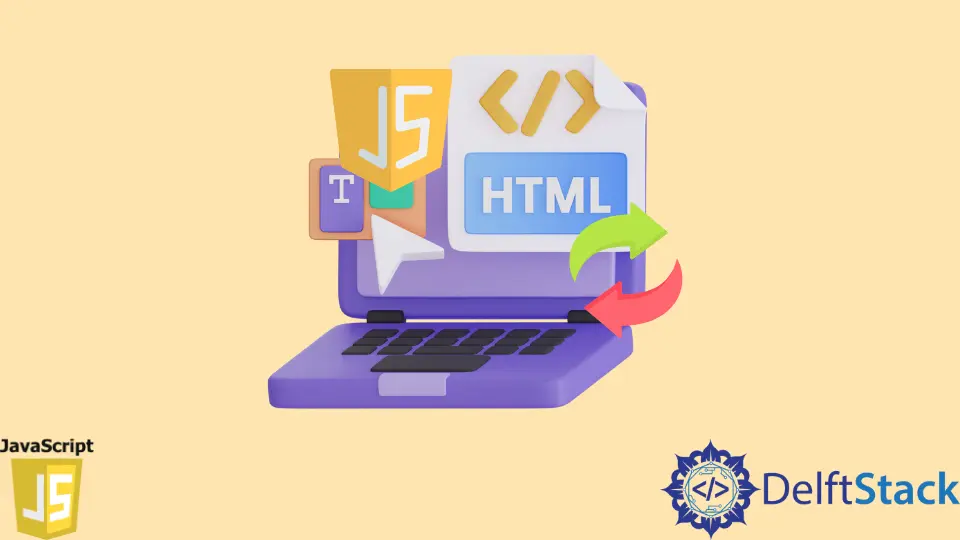
JavaScript is a powerful tool that breathes life into static web pages, enabling developers to create dynamic and interactive user experiences. One of the key features that facilitate this dynamism is the innerHTML
property. By manipulating the innerHTML
of an element, you can change the content displayed on a webpage in real time, making it adaptable to user interactions and other events.
In this article, we will explore how to effectively use JavaScript to change innerHTML
, providing practical examples and explanations to help you master this essential skill. Whether you’re building a landing page or enhancing an existing one, understanding how to utilize innerHTML
can significantly enhance your web development capabilities.
Understanding innerHTML
Before diving into the practical applications of innerHTML
, it’s essential to understand what it is. The innerHTML
property of an HTML element allows you to get or set the HTML content contained within that element. This means you can easily change text, images, and even entire HTML structures dynamically.
For example, if you have a <div>
that displays user comments, you can use JavaScript to update that <div>
with new comments without refreshing the page. This capability is crucial for creating seamless user experiences on modern websites.
Changing innerHTML with JavaScript
Method 1: Using getElementById
One of the most common ways to change the innerHTML
of an element is by using the getElementById
method. This method allows you to select an element by its unique ID and then modify its content.
Here’s a simple example:
document.getElementById("myElement").innerHTML = "Hello, World!";
In this code snippet, we are targeting an element with the ID of myElement
and changing its innerHTML
to “Hello, World!”. This is a straightforward approach and works well for elements that have unique IDs.
The getElementById
method is efficient and widely used due to its simplicity. It’s particularly useful when you know the specific element you want to manipulate. This method is also supported in all major browsers, ensuring compatibility across different platforms.
Output:
Hello, World!
Method 2: Using querySelector
Another powerful method to change innerHTML
is by using the querySelector
method. This method allows you to select elements using CSS selectors, providing greater flexibility compared to getElementById
.
Here’s how you can use it:
document.querySelector(".myClass").innerHTML = "<strong>Welcome to my website!</strong>";
In this example, we are selecting the first element with the class name myClass
and setting its innerHTML
to include bold text. The querySelector
method is particularly useful when you want to select elements based on classes, attributes, or even hierarchical structures.
The flexibility of querySelector
makes it a favorite among developers, especially for complex DOM manipulations. It allows for precise targeting of elements, making it easier to implement dynamic content changes.
Output:
Welcome to my website!
Method 3: Using addEventListener
Changing the innerHTML
dynamically in response to user actions is another common use case. You can achieve this by using the addEventListener
method to listen for events like clicks.
Here’s an example:
document.getElementById("myButton").addEventListener("click", function() {
document.getElementById("myElement").innerHTML = "You clicked the button!";
});
In this code, we are adding a click event listener to a button with the ID of myButton
. When the button is clicked, the innerHTML
of myElement
is updated to notify the user that the button was clicked. This method enhances interactivity on your webpage, engaging users and making their experience more enjoyable.
Using addEventListener
is a best practice in modern JavaScript development. It allows for cleaner code and better separation of concerns, keeping your event handling logic separate from your HTML structure.
Output:
You clicked the button!
Conclusion
Manipulating innerHTML
using JavaScript is an essential skill for any web developer. By understanding how to effectively use methods like getElementById
, querySelector
, and addEventListener
, you can create dynamic and interactive web pages that respond to user actions in real time. As you become more familiar with these techniques, you’ll find that the possibilities for enhancing user experience are virtually limitless. Whether you’re building a simple landing page or a complex web application, mastering innerHTML
will undoubtedly elevate your web development game.
FAQ
-
What is innerHTML in JavaScript?
innerHTML is a property that allows you to get or set the HTML content of an element. -
How do I change the innerHTML of an element?
You can change innerHTML by selecting an element and assigning new content to its innerHTML property.
-
Can I use innerHTML to insert HTML tags?
Yes, you can use innerHTML to insert HTML tags, which will be rendered by the browser. -
Is using innerHTML safe from XSS attacks?
Using innerHTML can expose your application to XSS attacks if user input is not properly sanitized. -
What are some alternatives to innerHTML?
Alternatives include using textContent for plain text or creating elements with methods like createElement and appendChild.