How to Change Form Action in JavaScript
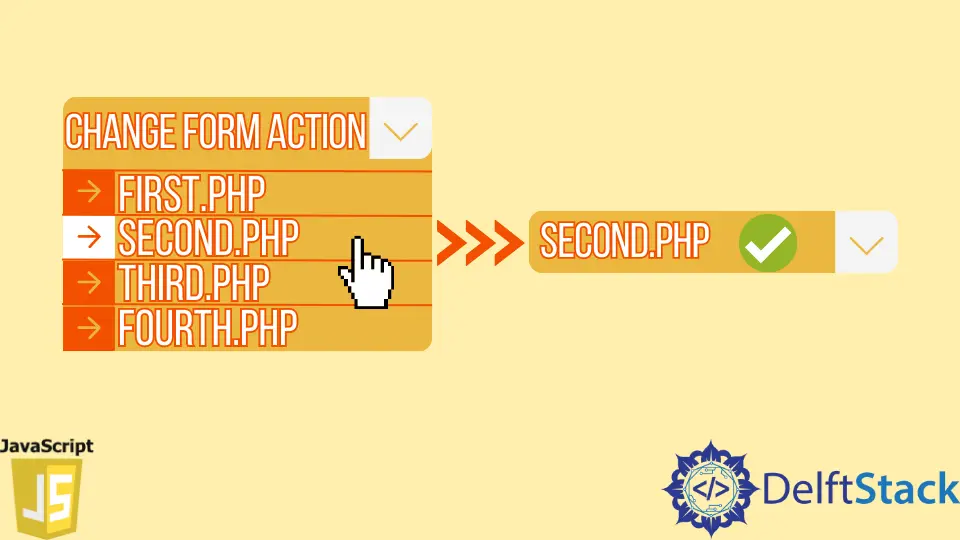
The action
attribute of HTML form
is an inevitable component in the form activities. Other than the post
and the get
method, these certain attributes ensure the path where the submitted data is meant to be stored for further usage.
Here, we will examine one example that will define the basic structure for the action change mechanism.
Change Form Action Based on onclick
Methods in JavaScript
In this case, we will initially state a form
with the necessary id
and method
as the post
and not initialize any action
in the HTML structure.
Then, a basic select-option
field is where we will work on, and our script tag will set an alert
that will notify us each time the option is altered.
When we have filled in our necessary information on this page, we can store the information in the preferred view or portal. This is where the submit
plays a role in directing the data pack to a different path via the new set action
.
Code Snippet:
<!DOCTYPE html>
<html>
<body>
<form id="form_id" method="post" name="myform">
<label>Form Action :</label>
<select id="form_action" onChange="select_change()" >
<option value="">--- Set Form Action ---</option>
<option value="first.php">first.php</option>
<option value="second.php">second.php</option>
<option value="third.php">third.php</option>
</select>
<input type="button" value="Submit" onclick="myfunction()"/>
</form>
<script>
function select_change() {
var index = document.getElementById("form_action").selectedIndex;
var option = document.getElementsByTagName("option")[index].value;
alert("Form action changed to " + option);
}
function myfunction() {
if (validation()) {
var x = document.getElementById("form_action").selectedIndex;
var action = document.getElementsByTagName("option")[x].value;
if (action !== "") {
document.getElementById("form_id").action = action;
document.getElementById("form_id").submit();
} else {
alert("Set form action");
}
}
}
</script>
</body>
</html>
Output: