How to Change the Background Color in JavaScript
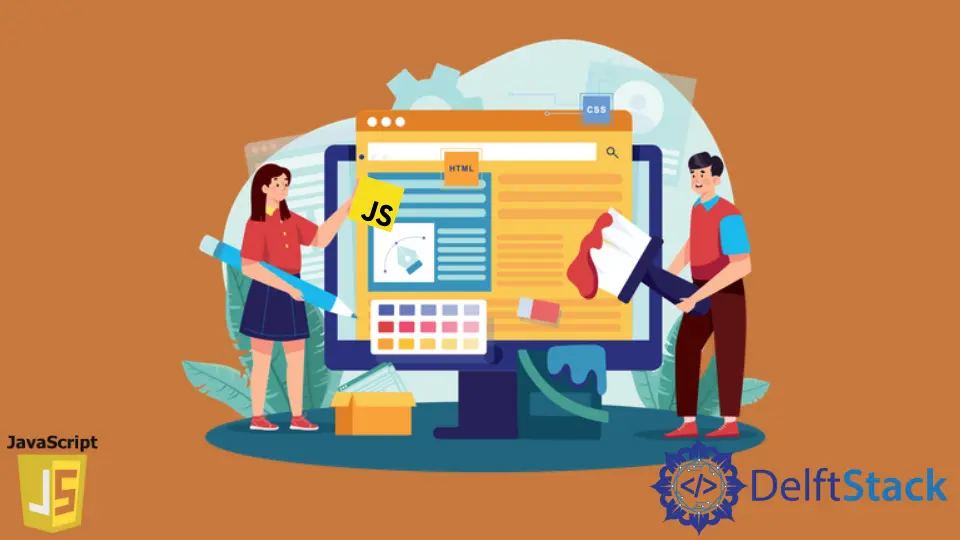
This tutorial will discuss how to change the background color using the backgroundColor
property in JavaScript.
Change the Background Color Using the backgroundColor
Property in JavaScript
We can change the background color using the backgroundColor
property in JavaScript. To use this property, you need to get the element whose background color you want to change, and then you can use the backgroundColor
property to set the background color.
For example, let’s create a page using HTML and change the background color of the body to green using the backgroundColor
property. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<script type="text/javascript">
document.body.style.backgroundColor = 'green';
</script>
</body>
</html>
You can also get the element with the id or name of the class. To get an element using its id, you can use the getElementById()
function. To get an element using its class, you can use the getElementsByClassName()
function.
For example, let’s get an element by its id and change its background color using the backgroundColor
property. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<p id='myID'>
Hello Wold
</p>
<script type="text/javascript">
document.getElementById('myID').style.backgroundColor = 'green';
</script>
</body>
</html>
The code above will only change the background color of the element with id myID
and not the whole body section. You also change the background color of an element using the color code of different colors instead of changing it using the color name.
Let’s write a code to change the background color of a text using a button. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<button onClick="Mycolor()">Change Color</button>
<div id="myID">Hello World</div>
<script type='text/javascript'>
function Mycolor() {
var element = document.getElementById("myID");
element.style.backgroundColor='#900';
}
</script>
</body>
</html>
In this code, you will see a button and a text. When you press the button, the background color of the text will change according to the given color.