How to Create Callback Function With Parameters in JavaScript
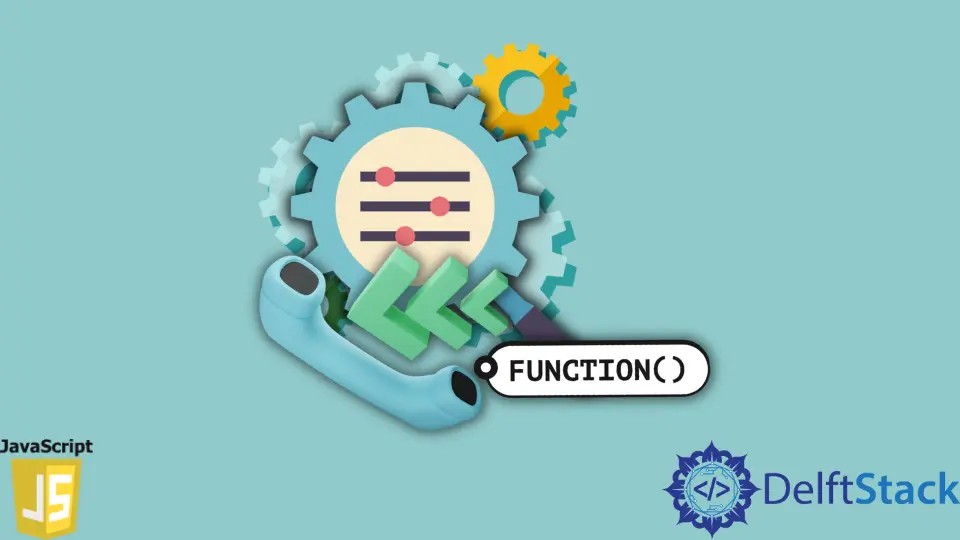
In JavaScript, when we pass a function to another function as a parameter, it is called a callback
function.
The function takes another function as a parameter and calls it inside.
A callback
function makes sure that a function will not run until the task completes.
Create a Callback
Function by Passing a Function as a Parameters in JavaScript
We will create a callback
function by passing the function as a parameter to another function. We call the function back right after the task completes.
We will make a function named sayName
. Then we create a callback
function named sayHowAreYou
.
We want to pass another parameter in the callback
function by the formal argument n
.
function sayName(name, cb) {
console.log(`Hello ${name}`);
cb(name);
}
function sayHowAreYou(n) {
console.log('How are you? ' + n);
}
sayName('DelftStack', sayHowAreYou);
Output:
Hello DelftStack
How are you? DelftStack
When we call the first function, it will return Hello DelftStack
. Then we greeted how are you
, so we called the callback
function for that purpose.
When we had passed the callback
function parameter, it returned Hello DelftStack, How are you? DelftStack
.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn