How to Call Function by Name in JavaScript
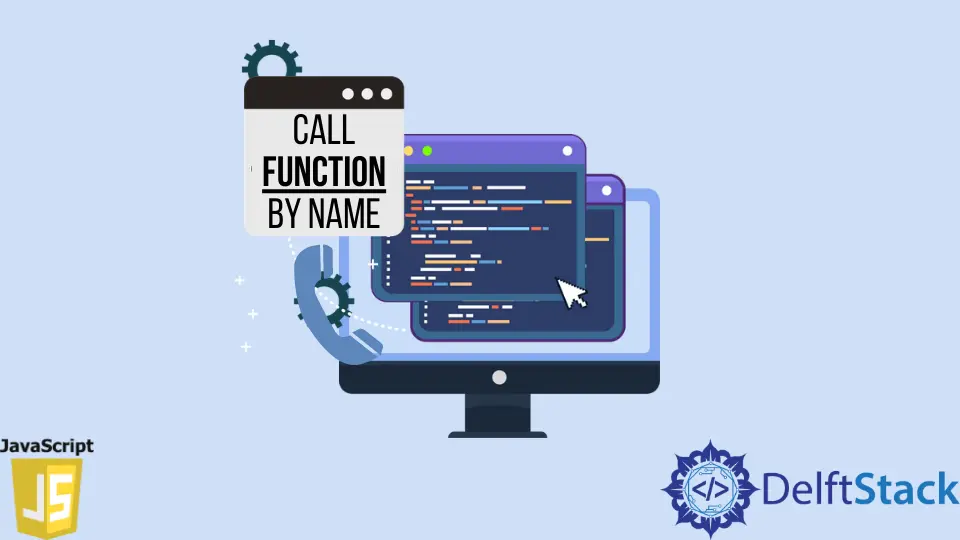
In JavaScript, sometimes it’s convenient to store function names in the string because we can use those strings to call the actual functions.
We can call a JavaScript function whose name is stored in a string variable using the eval()
method but is an old method.
We will use the window object
method to call a function.
Use the window object
Method to Call Function by Its Name in JavaScript
We created a function named changeColor()
. We stored that function in the string variable.
Now, we want to call that function we stored in the string. We need to click on the button.
In the string function, we pass parameter red. So, when we click on the button, the color of the text You called the Function
changes to red.
<!DOCTYPE html>
<html>
<head>
<title>
To call a function
</title>
</head>
<body>
<h1 style="color: Red">
Call function by name
</h1>
<b>
To call a function by its name stored in string variable in JavaScript.
</b>
<p>
Click on the button to call the function in the string.
</p>
<p class="example">
You called the function.
</p>
<button onclick="evaluateFunction()">
Click Here
</button>
<script type="text/javascript">
function changeColor(color) {
document.querySelector('.example').style
= `color: ${color}`;
}
function evaluateFunction() {
stringFunction = "changeColor";
param = 'red';
window[stringFunction](param);
}
</script>
</body>
</html>
In this way, we call the string function by using the window[stringFunction](param)
method.
Output:
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn