Bubble Sort in JavaScript
Harshit Jindal
Oct 12, 2023
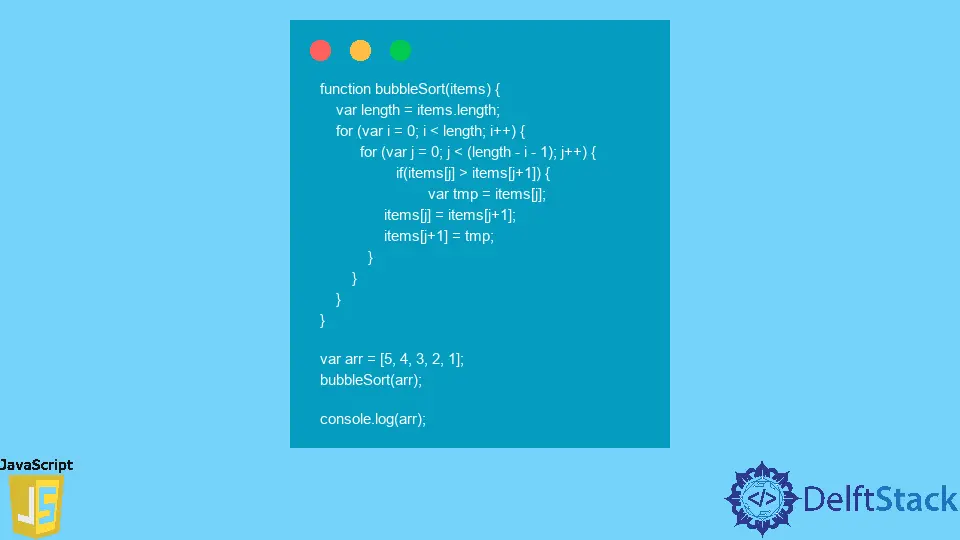
This tutorial teaches how to sort arrays using bubble sort in JavaScript.
Note
If you don’t know what bubble sort is, then please go through the Bubble Sort article first.
Bubble sort is a simple sorting algorithm. It works by repeated comparison of adjacent elements and swapping them if they are in the wrong order. The repeated comparisons bubble up the smallest/largest element towards the end of the array, and hence this algorithm is named bubble sort. Although inefficient, it still represents the foundation for sorting algorithms.
JavaScript Bubble Sort Implementation
function bubbleSort(items) {
var length = items.length;
for (var i = 0; i < length; i++) {
for (var j = 0; j < (length - i - 1); j++) {
if (items[j] > items[j + 1]) {
var tmp = items[j];
items[j] = items[j + 1];
items[j + 1] = tmp;
}
}
}
}
var arr = [5, 4, 3, 2, 1];
bubbleSort(arr);
console.log(arr);
Output:
[1, 2, 3, 4, 5]
Author: Harshit Jindal
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn