JavaScript Boolean Function
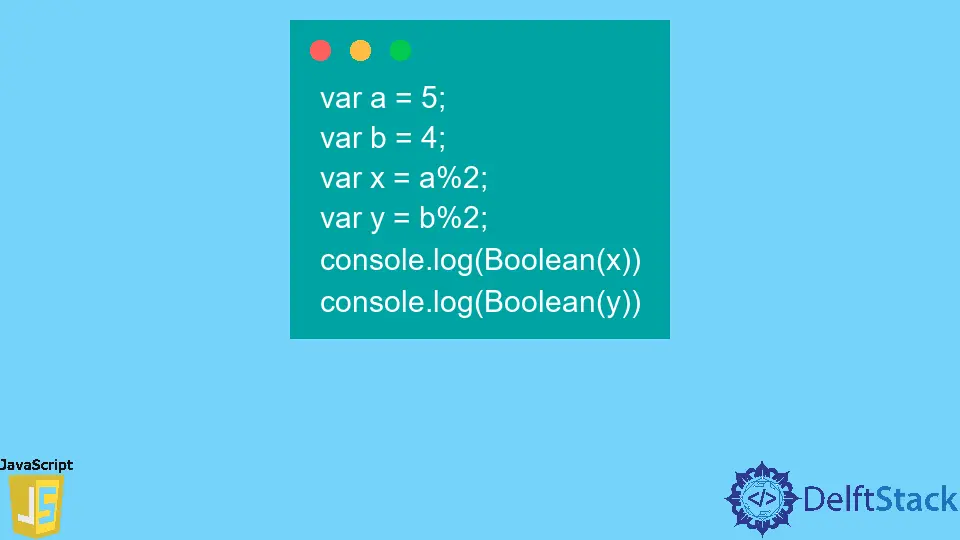
In the world of programming, data types play a crucial role in how we handle information. One such fundamental type in JavaScript is the Boolean data type, which operates exclusively with two values: true and false. The Boolean function in JavaScript is essential for making decisions, controlling flow, and determining the truthiness of expressions. Unlike numerical values like 0 and 1, Booleans are distinct and self-defining, allowing developers to create more intuitive and readable code.
In this article, we will explore the JavaScript Boolean function, its significance, and practical examples to illustrate its usage.
What is a Boolean in JavaScript?
A Boolean represents a logical entity that can have two values: true or false. This data type is particularly useful in control structures such as if statements, loops, and logical operations. When you use a Boolean, you are essentially making a statement about a condition that can either be met (true) or not met (false).
The Boolean function can be explicitly called to convert a value to a Boolean. For instance, when passing a non-Boolean value, JavaScript will evaluate it and return true or false based on its truthiness. Understanding how this works is essential for effective coding in JavaScript.
Using the Boolean Function
The Boolean function can be invoked in various ways. Here are a few examples that demonstrate how to use it effectively:
Example 1: Basic Usage of the Boolean Function
console.log(Boolean(1));
console.log(Boolean(0));
console.log(Boolean("Hello"));
console.log(Boolean(""));
console.log(Boolean(null));
Output:
true
false
true
false
false
In this example, we are using the Boolean function to evaluate different types of values. The number 1 evaluates to true, while 0 evaluates to false. Similarly, non-empty strings are considered true, while an empty string evaluates to false. The null value also returns false. This demonstrates how the Boolean function can be used to determine the truthiness of various expressions.
Example 2: Conditional Statements with Booleans
let isRaining = false;
if (Boolean(isRaining)) {
console.log("Take an umbrella.");
} else {
console.log("No need for an umbrella.");
}
Output:
No need for an umbrella.
In this code snippet, we define a variable called isRaining and set it to false. The if statement checks the Boolean value of isRaining. Since it is false, the else block executes, and we see the message “No need for an umbrella.” This example highlights how Booleans control the flow of logic in our code.
Example 3: Logical Operations with Booleans
let hasKey = true;
let isDoorLocked = false;
if (hasKey && !isDoorLocked) {
console.log("You can enter the house.");
} else {
console.log("You cannot enter the house.");
}
Output:
You can enter the house.
Here, we are using logical operators to combine Boolean values. The hasKey variable is true, and isDoorLocked is false. The expression checks if hasKey is true and isDoorLocked is false. Since both conditions are met, the message “You can enter the house.” is displayed. This example illustrates how Boolean logic can be used to create complex conditions.
Conclusion
The JavaScript Boolean function is a powerful tool that allows developers to make logical decisions within their code. By understanding how to use true and false values effectively, you can create more robust and readable applications. Whether you are controlling flow with if statements or performing logical operations, the Boolean function is an essential part of JavaScript programming. As you continue to explore JavaScript, remember the importance of the Boolean data type in shaping your code’s logic.
FAQ
-
What is a Boolean in JavaScript?
A Boolean in JavaScript is a data type that can hold one of two values: true or false. -
How does the Boolean function work?
The Boolean function converts a value to a Boolean, evaluating its truthiness based on certain rules.
-
Can I use Boolean values in conditional statements?
Yes, Boolean values are commonly used in conditional statements to control the flow of logic in your code. -
What values evaluate to false in JavaScript?
Values such as 0, null, undefined, NaN, and an empty string ("") evaluate to false. -
How can I combine Boolean values?
You can combine Boolean values using logical operators like AND (&&), OR (||), and NOT (!).