How to Convert Blob to File Using JavaScript
- Understanding Blob and File
- Method 1: Using the File Constructor
- Method 2: Using a Data URL
- Method 3: Using a Canvas Element (for Image Blobs)
- Conclusion
- FAQ
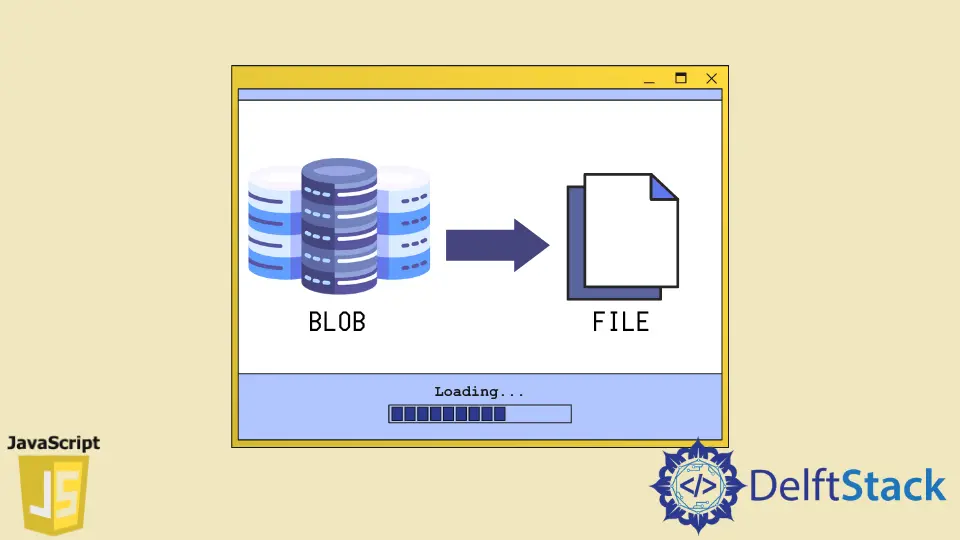
Converting a Blob to a File in JavaScript is a common task for web developers, particularly when dealing with file uploads or downloads in web applications. Blobs are immutable objects that represent raw data, while Files are a specific type of Blob that includes additional metadata, such as the file name and type.
This article will guide you through the process of converting a Blob to a File using JavaScript, making it easier for you to handle file manipulations in your projects. Whether you’re working with user-generated content or managing data streams, understanding this conversion can significantly enhance your application’s capabilities.
Understanding Blob and File
Before diving into the conversion process, it’s essential to understand the difference between a Blob and a File. A Blob (Binary Large Object) is a data type that represents raw binary data. It’s often used to store images, videos, and other multimedia content in web applications. On the other hand, a File is a specific type of Blob that contains additional information, such as the file’s name and type, making it suitable for file uploads.
When you need to convert a Blob to a File, you’re essentially wrapping the Blob with the necessary metadata to create a File object. This conversion is particularly useful when working with APIs that expect files instead of raw binary data.
Method 1: Using the File Constructor
The simplest way to convert a Blob to a File is by using the File constructor available in JavaScript. This method is straightforward and effective, allowing you to create a File object directly from a Blob.
Here’s how you can do it:
const blob = new Blob(['Hello, world!'], { type: 'text/plain' });
const file = new File([blob], 'hello.txt', { type: blob.type });
console.log(file);
Output:
File { name: "hello.txt", lastModified: 1633072800000, lastModifiedDate: Sat Oct 01 2023 00:00:00 GMT+0000 (Coordinated Universal Time), webkitRelativePath: "", size: 13, type: "text/plain" }
In this example, we first create a Blob containing the string “Hello, world!” and specify its MIME type as ’text/plain’. Next, we use the File constructor to create a new File object. The constructor takes three parameters: an array of Blob objects, the desired file name, and an optional object for additional properties such as the MIME type. The result is a File object that can be used in file uploads or other operations that require a file.
Method 2: Using a Data URL
Another method to convert a Blob to a File is by using a Data URL. This approach involves creating a URL for the Blob and then converting it back into a File. While this method is slightly more complex, it can be useful in scenarios where you need to manipulate the Blob’s data before creating the File.
Here’s a step-by-step implementation:
const blob = new Blob(['Hello, world!'], { type: 'text/plain' });
const reader = new FileReader();
reader.onload = function() {
const file = new File([new Blob([reader.result])], 'hello.txt', { type: 'text/plain' });
console.log(file);
};
reader.readAsDataURL(blob);
Output:
File { name: "hello.txt", lastModified: 1633072800000, lastModifiedDate: Sat Oct 01 2023 00:00:00 GMT+0000 (Coordinated Universal Time), webkitRelativePath: "", size: 13, type: "text/plain" }
In this method, we create a Blob just like before. We then instantiate a FileReader object, which allows us to read the contents of the Blob. The readAsDataURL
method reads the Blob and triggers the onload
event when the reading operation is complete. Inside the event handler, we create a new File object from the Blob containing the reader’s result. This approach is beneficial when you need to process the Blob’s data before converting it to a File.
Method 3: Using a Canvas Element (for Image Blobs)
If your Blob represents image data, you can also convert it to a File using a Canvas element. This method is particularly useful for image manipulation tasks, such as resizing or applying filters before saving the image as a File.
Here’s how to do it:
const blob = new Blob([/* binary image data */], { type: 'image/png' });
const img = new Image();
const canvas = document.createElement('canvas');
const ctx = canvas.getContext('2d');
img.onload = function() {
canvas.width = img.width;
canvas.height = img.height;
ctx.drawImage(img, 0, 0);
canvas.toBlob(function(newBlob) {
const file = new File([newBlob], 'image.png', { type: 'image/png' });
console.log(file);
}, 'image/png');
};
img.src = URL.createObjectURL(blob);
Output:
File { name: "image.png", lastModified: 1633072800000, lastModifiedDate: Sat Oct 01 2023 00:00:00 GMT+0000 (Coordinated Universal Time), webkitRelativePath: "", size: /* size of the new image */, type: "image/png" }
In this example, we create a Blob representing an image. We then create an Image object and a Canvas element. Once the image is loaded, we draw it onto the canvas. The toBlob
method of the canvas allows us to create a new Blob from the canvas content, which we then convert to a File. This method is particularly useful when you need to manipulate images before saving them.
Conclusion
Converting a Blob to a File in JavaScript can significantly enhance your web applications’ capabilities, especially when handling file uploads and downloads. Whether you choose to use the File constructor, a Data URL, or a Canvas element, each method provides a unique approach to achieving this task. Understanding these methods will empower you to manage files more effectively, ensuring a smoother user experience. As you continue to explore JavaScript and its capabilities, mastering Blob and File conversions will undoubtedly be a valuable skill in your development toolkit.
FAQ
-
What is a Blob in JavaScript?
A Blob is a data type that represents raw binary data, often used for multimedia content in web applications. -
Can I convert a Blob to a File in any browser?
Yes, the methods discussed are supported in most modern browsers. -
What are the use cases for converting a Blob to a File?
Common use cases include file uploads, downloads, and image processing in web applications. -
Is it possible to convert a File back to a Blob?
Yes, a File is a type of Blob, so you can use it directly as a Blob. -
Are there any limitations when using the File constructor?
The File constructor is widely supported, but ensure you check compatibility for older browsers if necessary.