BigDecimal in JavaScript
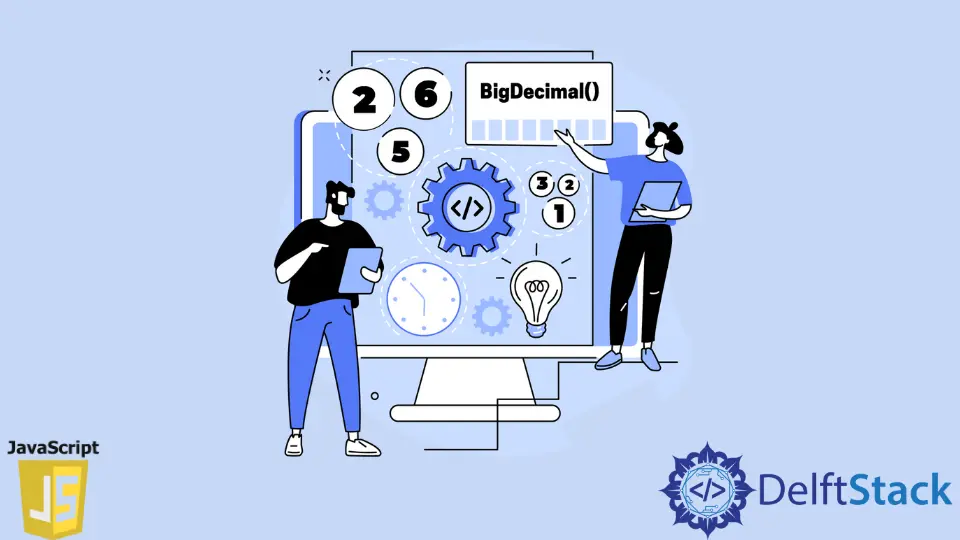
This article will discuss the uses of BigDecimal
in JavaScript.
Use of BigDecimal
in JavaScript
Before you work on how BigDecimal
works in JavaScript, you must know about BigDecimal
. A BigDecimal
consists of an unscaled arbitrary precision integer value with a 32-bit integer scale.
If the scale is zero or positive, it is the number of digits to the right of the decimal point. If the integer is negative, the unscaled value is multiplied by ten to the power of the scale’s negation.
BigDecimal
determines equality and hash codes using the decimal representation of binary floating-point integers. This produces different results than conversion between Long and Double numbers, which uses the precise form.
Note that you must install the npm
package of BigDecimal
before running the code.
Save the below code as a js
extension file.
let bDecimal = require('bigdecimal');
let num = new bDecimal.BigInteger('123456abcdefghijklmn7890', 25);
console.log('num is ' + num);
let newD = new bDecimal.BigDecimal(num);
let k = new bDecimal.BigDecimal('4567890.12345612345678901234567890123');
console.log('newD * k = ' + newD.multiply(k));
let two = new bDecimal.BigDecimal('2');
console.log('Average = ' + newD.add(k).divide(two));
You can see different method like as multiply()
, add()
, divide()
. The add()
method add one BigDecimal
object value to other where newD
is a BigDecimal
object, newD
is added to k
.
Whereas the multiply()
method multiplies one BigDecimal
object value to another, the divide()
method divides the one BigDecimal
value by another.
When converting a Double or Float to a BigDecimal
, caution must be given since the binary fraction representation of the Double and Float does not simply transfer to a decimal representation.
Output:
BigDecimal
is ideal for financial data arithmetic or anything that exceeds the JavaScript Integer (IEEE-754 float) type. Decimal was not included in the new ECMAScript standards.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn