JavaScript Average Function
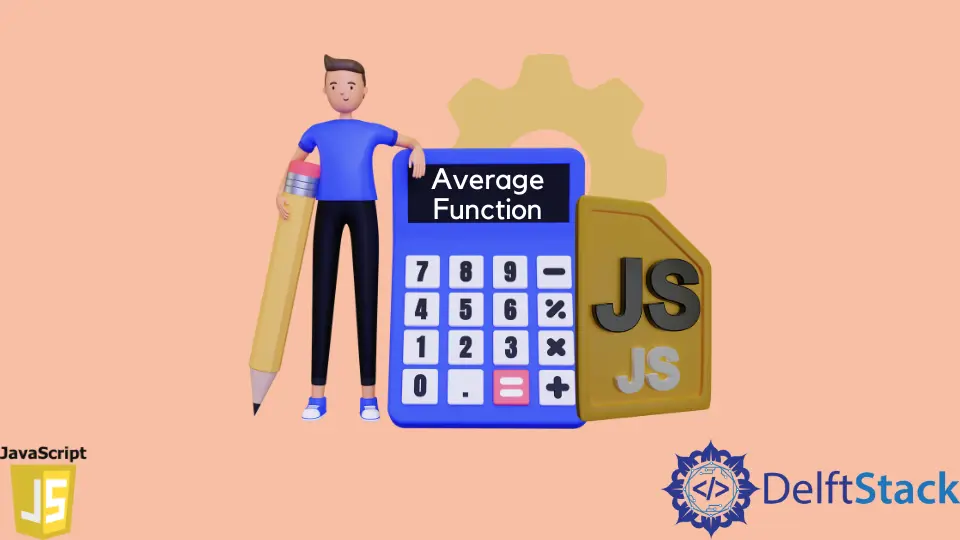
This article will discuss making a function to calculate the average of an array using a loop in JavaScript.
Make a Function to Calculate the Average of an Array Using a Loop in JavaScript
There is no predefined function available in JavaScript to calculate the average of an array. We can make the function to get the average of an array using the average formula and a loop. To find the average of an array, we have to find the sum of all the elements present in an array using a loop and then divide the sum with the number of elements present in the array, which we can find using the length
function. For example, let’s create the function to calculate the average of a given array and test it with an array and show the result of the average on the console. See the code below.
function ArrayAvg(myArray) {
var i = 0, summ = 0, ArrayLen = myArray.length;
while (i < ArrayLen) {
summ = summ + myArray[i++];
}
return summ / ArrayLen;
}
var myArray = [1, 5, 2, 3, 7];
var a = ArrayAvg(myArray);
console.log(a)
Output:
3.6
Using this function, you can also find the average of an array containing floating-point values.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript