How to Append Data to Div in JavaScript
-
Use
innerHTML
to Append Data to Div in JavaScript -
Use
appendChild
to Append Data to Div in JavaScript -
Use
insertAdjacentHTML()
to Append Data to Div in JavaScript -
Use jQuery’s
append()
to Append Data to Div
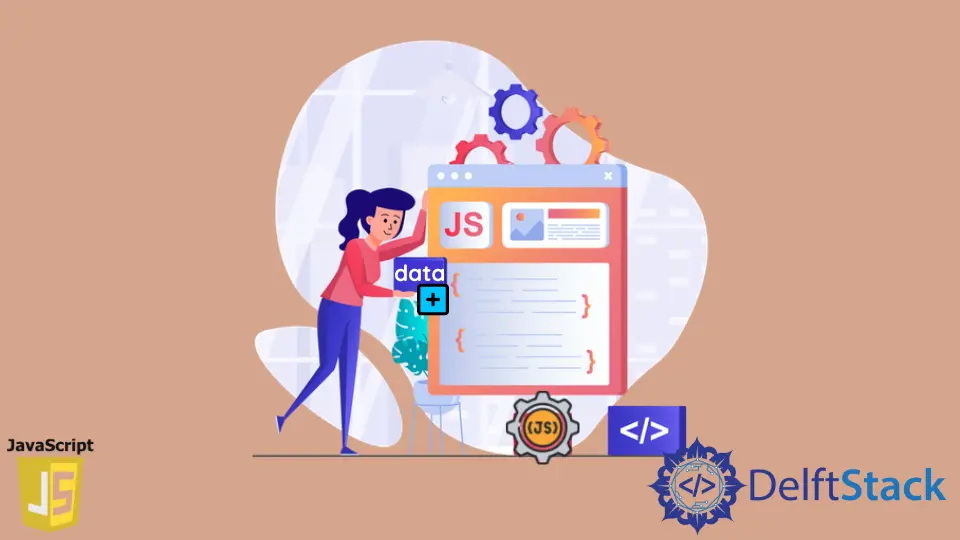
This tutorial introduces how to append data to div using JavaScript.
Use innerHTML
to Append Data to Div in JavaScript
In this method, we first select the div
by using one of the selectors i.e. getElementById
, getElementByTag
, querySelector
, etc. We then append the data to the innerHTML
attribute of div. This method has one disadvantage: it removes all the existing event listeners on the selected div
. It will also destroy all nodes contained inside and replace them with new ones. All references to the elements will be lost.
var targetDiv = document.getElementById('ID');
targetDiv.innerHTML += 'data that you want to add';
Use appendChild
to Append Data to Div in JavaScript
Like the previous method, we first select the div
by using one of the selectors. But instead of innerHTML
, we use appendChild
because of the problems discussed in the previous method. Before appending a child, we have to create the text node.
var targetDiv = document.getElementById('ID');
var content = document.createTextNode('data that you want to add');
targetDiv.appendChild(content);
Use insertAdjacentHTML()
to Append Data to Div in JavaScript
Like the second method, this method is a better alternative to the first method. insertAdjacentHTML()
doesn’t reparse the element, and it also doesn’t interfere with the existing elements inside. It is much faster than innerHTML
. It also helps us specify the position at which to insert the data. Like previous methods, we first select the div
, and then we use insertAdjacentHTML()
to add the data at the required position.
var targetDiv = document.getElementById('ID');
targetDiv.insertAdjacentHTML('afterend', 'data that you want to add');
Use jQuery’s append()
to Append Data to Div
The append function takes the input of the content that needs to be added and can be called directly on a tag using jQuery syntax. This method also has all the drawbacks of the innerHTML
method.
$('.divd').append('<p>Test</p>');
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn