How to Append Array to Another in JavaScript
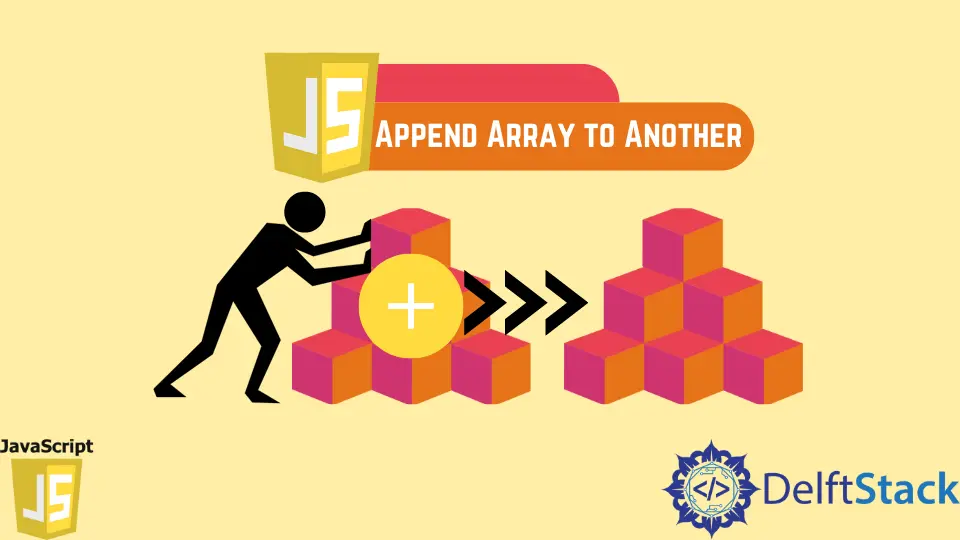
Appending one array to another in JavaScript is a common task that developers encounter frequently. Whether you’re working on a simple project or a complex application, managing arrays efficiently can make a significant difference in your code’s performance and readability. JavaScript provides several methods to achieve this, with push()
and concat()
being the most popular.
In this article, we’ll explore how to append arrays using these methods, providing clear examples and explanations along the way. By the end, you’ll have a solid understanding of how to manipulate arrays in JavaScript, making your coding experience smoother and more efficient.
Using the push()
Method
The push()
method is a straightforward way to append elements to an existing array. This method modifies the original array by adding new items to its end. If you want to append another array to an existing array, you can use the spread operator to achieve this effectively.
Here’s how it works:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
array1.push(...array2);
console.log(array1);
Output:
[1, 2, 3, 4, 5, 6]
In this example, we start with two arrays: array1
and array2
. By using the spread operator (...
), we can unpack the elements of array2
and push them into array1
. The push()
method modifies array1
directly, resulting in a single array containing all the elements from both arrays. This method is particularly useful when you want to keep the original array intact while adding new elements.
The push()
method is efficient and works well for adding individual elements or appending arrays. However, remember that it alters the original array, which may not always be desirable. If you need to maintain the original array without modifications, consider using the concat()
method instead.
Using the concat()
Method
The concat()
method is another powerful way to append arrays in JavaScript. Unlike push()
, concat()
does not modify the original arrays but instead returns a new array that combines the elements of the arrays you provide.
Here’s how to use concat()
:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
let combinedArray = array1.concat(array2);
console.log(combinedArray);
Output:
[1, 2, 3, 4, 5, 6]
In this example, array1
and array2
are concatenated using the concat()
method. The result is stored in a new variable called combinedArray
. This method is particularly useful when you want to create a new array without altering the originals.
The concat()
method can also take multiple arrays or values as arguments, making it versatile for various situations. For instance, you can append several arrays at once:
let array3 = [7, 8, 9];
let combinedMultiple = array1.concat(array2, array3);
console.log(combinedMultiple);
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
In this case, combinedMultiple
contains elements from all three arrays. The concat()
method is an excellent choice when you want to ensure that the original arrays remain unchanged while still combining their elements.
Conclusion
Appending arrays in JavaScript is a fundamental skill that can enhance your programming capabilities. Whether you choose to use the push()
method for its simplicity or the concat()
method for its non-destructive nature, understanding these techniques will empower you to manage data more effectively. Both methods have their unique advantages, so consider your specific needs when deciding which to use. With practice, you’ll find that working with arrays becomes second nature, allowing you to create more dynamic and responsive applications.
FAQ
-
What is the difference between push() and concat() in JavaScript?
push() modifies the original array by adding elements to its end, while concat() creates a new array without altering the original arrays. -
Can I append multiple arrays at once using concat()?
Yes, concat() allows you to append multiple arrays or values in a single call. -
Is the spread operator necessary when using push() to append an array?
Yes, the spread operator is needed to unpack the elements of the array you want to append. -
Can I use push() to append an array to another array without modifying the original?
No, push() modifies the original array. If you want to keep the original intact, use concat().
- Are there performance differences between push() and concat()?
While both methods are efficient, push() may be faster for adding individual elements, while concat() is better for combining multiple arrays without altering the originals.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript