How to Alert Yes No With the confirm() Function in JavaScript
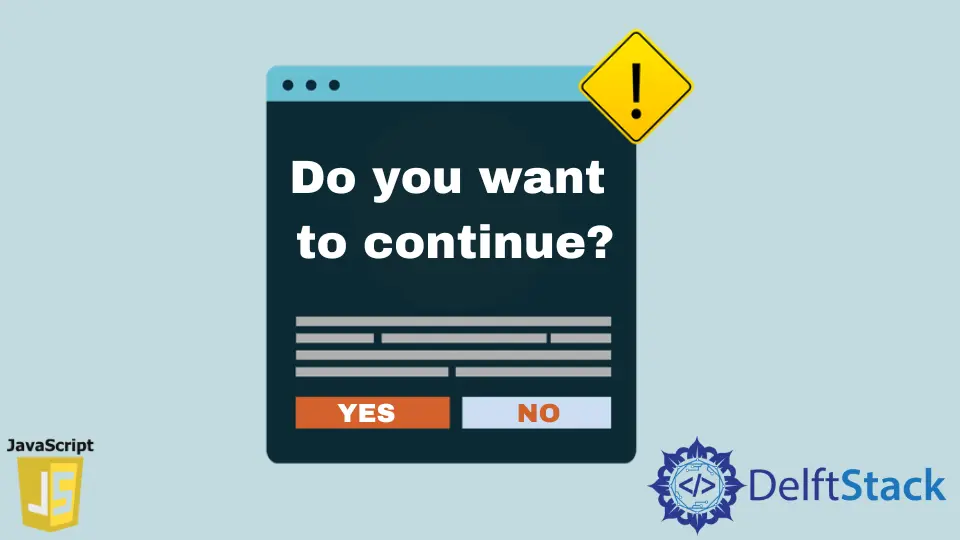
This tutorial discusses how to create a dialog using the confirm()
function in JavaScript.
Create a Dialog Using the confirm()
Function in JavaScript
To create a dialog, we can use the confirm()
function in JavaScript. This function asks the user for his opinion and returns true
or false
depending on the user’s choice. You can use the returned value to perform your desired function.
For example, let’s create a dialog with some text using the confirm()
function. See the below code.
var dialog = confirm('Save data?');
if (dialog) {
console.log('Data Saved')
} else {
console.log('Data Not Saved')
}
Output:
Data Saved
When you run the code above, a dialog will appear that will ask you if you want to save data or not. If you press ok
, then the output above will appear on the console.
The console.log()
function is used to print the text on the console. If the confirm()
function cause problems, you can also use the window.confirm()
function to avoid any problem.
Now, the dialog above will appear when you reload or open the page. Still, you can also attach it with a button or a link; this way, when a button or link is pressed, this dialog will appear. Otherwise, not.
For example, let’s use a button to ask the user for confirmation to save data. See the code below.
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<button type="button" onclick="if(confirm('Save Data?')){ console.log('Data Saved')};">SomeButton</button>
</body>
</html>
Output:
Data Saved
In the code above, we use a button to display the dialog, but you can also use a link or form to display it according to your requirements.