How to Alert New Line in JavaScript
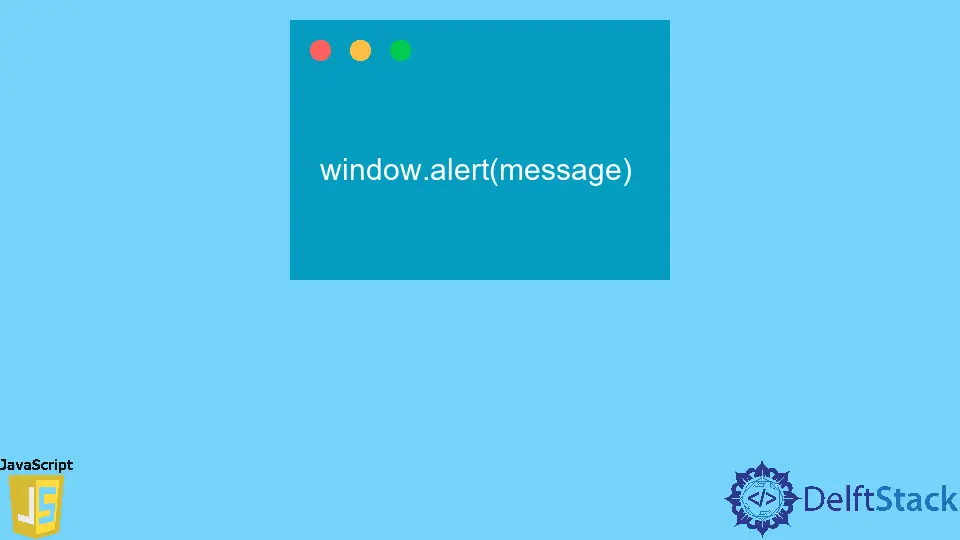
In today’s post, we’ll learn about adding new lines to the alert in JavaScript.
Add New Line in Alert in JavaScript
window.alert()
tells the browser to display a dialog with an optional message and wait for the user to close the dialog.
Under certain conditions, such as when the user switches between tabs, the browser may not display a dialog box or wait for the user to close the dialog box.
Syntax:
window.alert(message)
The alert
method takes a character string you want to display in the alarm dialog or an object converted to a character string and displayed.
Dialogs are modal windows that prevent the user from accessing the rest of the program interface until the dialog is closed. For this reason, you shouldn’t abuse any function that creates a dialog (or modal window).
Let’s look at the example below.
alert('Hello World!\nWelcome to my blog post.');
In the above example, we have given two messages as an input argument to the alert function. Both messages are separated using \n
, which means that the first message is displayed in the first row/line and another in the second row/line.
Try to run the above code in any browser that supports JavaScript, and it will show you the below result.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn