How to Add Minutes to Date in JavaScript
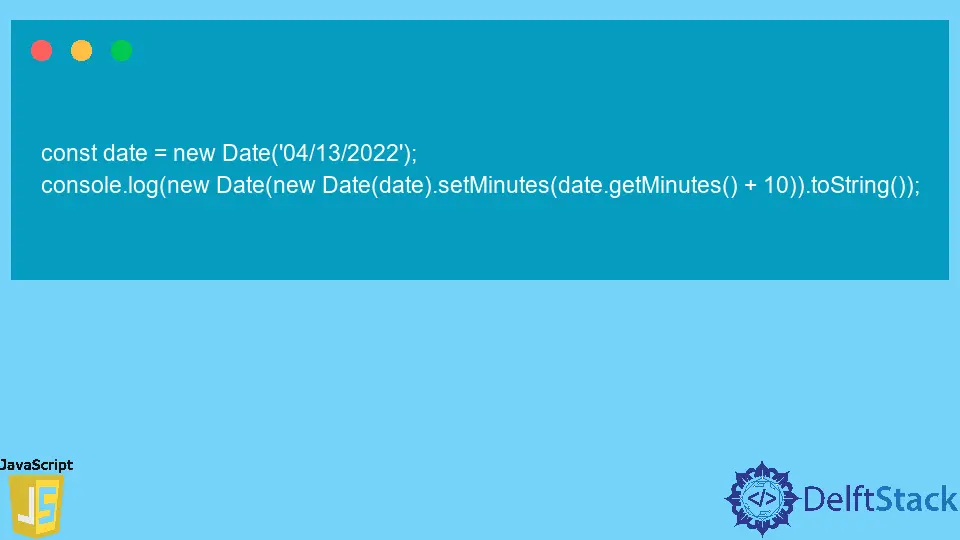
In today’s post, we’ll learn to modify the date
object and add minutes to the date
object in JavaScript.
Add Minutes to Date in JavaScript
JavaScript date
objects constitute a single factor in time in a platform-independent format. Date
object incorporates a number representing milliseconds since January 1, 1970, UTC
.
When a new Date()
is called, it returns a new Date
object, and when Date()
is called, it returns a string representation of the current date and time.
JavaScript provides various static methods like Date.now()
, Date.parse()
and Date.UTC()
. Some of the instance methods used to alter or access the Date
object are Date.prototype.getMinutes()
, Date.prototype.getSeconds()
, Date.prototype.setDay()
, Date.prototype.getMilliseconds()
, etc.
Find more information in the documentation for Date
.
The input date needs to be converted to the Date
objects to add the dynamic minutes to the existing Date
object. Let’s understand it with the following example.
const date = new Date('04/13/2022');
console.log(
new Date(new Date(date).setMinutes(date.getMinutes() + 10)).toString());
The example above has defined the date
variable containing the Date
object. To set the minutes, call the new
constructor with the input date
object and call the setMinutes
method to set the minutes to the date.
The getMinutes()
method returns an integer between 0 and 59, representing the minutes of the specified date based on local time. The setMinutes()
sets the minutes for a given date based on local time.
You can specify seconds and milliseconds as an argument to the setMinutes()
method.
secondsValue
and msValue
parameters are optional parameters. If you do not specify these parameters, values returned from getSeconds()
and getMilliseconds()
methods are used.
Several milliseconds are returned between January 1, 1970 00:00:00 UTC
and the updated date. The .toString()
method gets the date in string format.
Attempt to run the above code snippet in any browser that supports JavaScript; it will display this result.
"Wed Apr 13 2022 00:10:00 GMT+0530 (India Standard Time)"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn