How to Add ID to an HTML Element in JavaScript
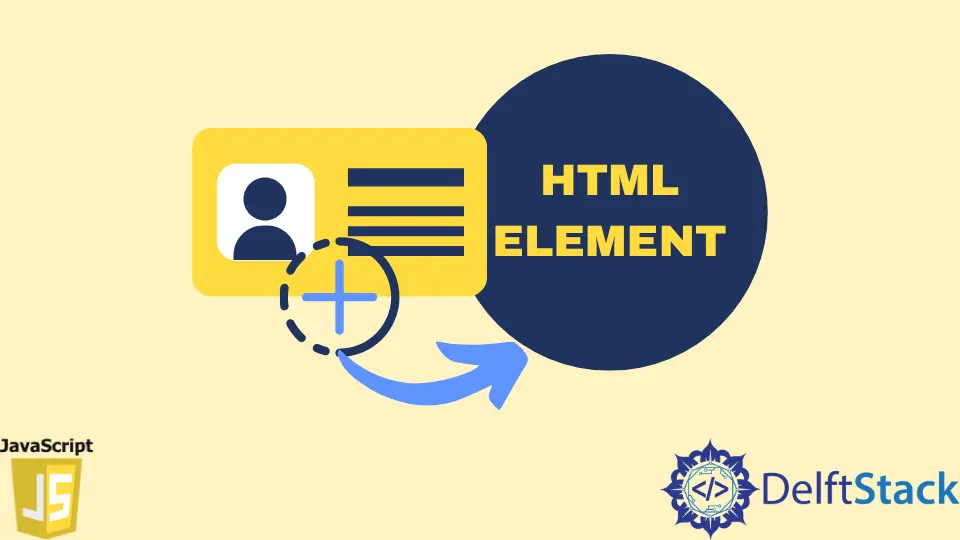
This article will show how to set an ID to an HTML element using JavaScript’s Element.id
property.
Add ID to an HTML Element Using the Element.id
Property in JavaScript
To add a unique ID to an HTML element whenever an element is created, we can use the id
property of the DOM API in JavaScript.
Here, we will create five div elements and add IDs dynamically to each. All the code we will write will only be inside the JavaScript file.
Inside JavaScript, we will create a for
loop that will run from zero to four. We will first create a div element at every iteration of this for loop using the createElement()
method.
Then we will store this div which we have created inside a variable ele
. It is a local variable whose value will be different at every iteration.
for (var i = 0; i < 5; i++) {
var ele = document.createElement('div');
ele.innerHTML = 'Hello';
ele.id = i + 1;
document.body.append(ele);
}
After creating the element, we will add a text inside it to see the div element on the screen. Using the id
property, we will set the ID of the ele
.
Here, we assign the variable i
to the ele.id
. Since the value of variable i
starts with 0, we add 1 to this variable and assign it to the id
property.
Finally, we will append ele
, which we have created to the body tag using the append()
method.
Here, we have set the IDs to the div elements dynamically with the help of a for
loop and the Element.id
method.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn