JavaScript 2D Array
- Understanding 2D Arrays in JavaScript
- Method 1: Using Array Literals
- Method 2: Using Nested Loops
- Method 3: Using Array.from()
- Conclusion
- FAQ
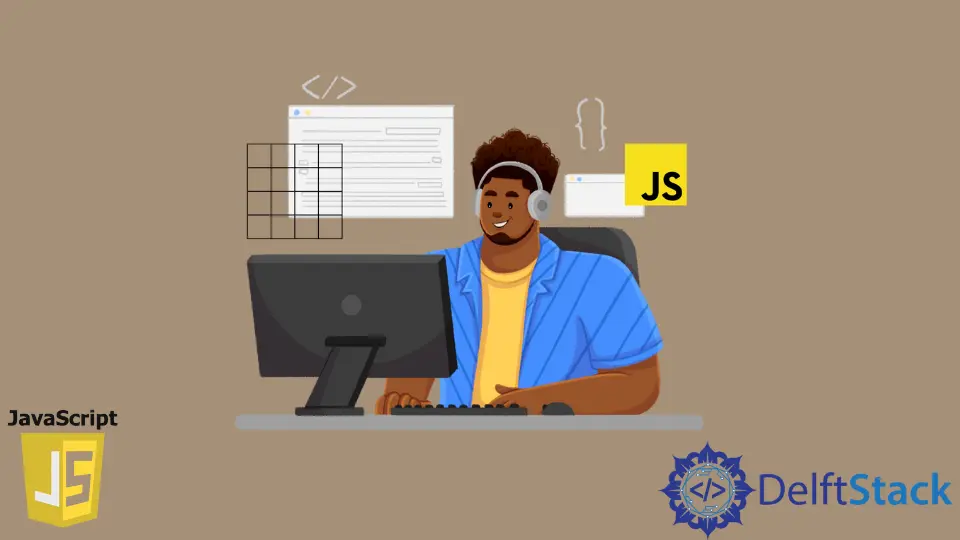
When diving into JavaScript, one of the fundamental concepts to grasp is the 2D array. A two-dimensional array is essentially an array of arrays, allowing you to store data in a grid-like structure. This can be particularly useful for representing matrices, game boards, or any scenario where you need to manage data in rows and columns.
In this tutorial, we will explore how to declare a two-dimensional array in JavaScript, providing you with clear examples and explanations. Whether you’re a beginner or looking to refresh your skills, this guide will help you understand the ins and outs of 2D arrays in JavaScript.
Understanding 2D Arrays in JavaScript
Before we dive into the declaration of 2D arrays, it’s important to understand what they are. A 2D array can be visualized as a table with rows and columns. Each element in a 2D array can be accessed using two indices: the first for the row and the second for the column. This structure is particularly handy when you need to handle complex data sets or perform operations like matrix multiplication.
Declaring a 2D Array
To declare a two-dimensional array in JavaScript, you can use either of the following methods. Let’s explore them in detail.
Method 1: Using Array Literals
One of the simplest ways to create a 2D array is by using array literals. This method involves defining the array directly with nested arrays. Here’s how you can do it:
let twoDArray = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
In this example, we created a 2D array named twoDArray
containing three rows and three columns. Each inner array represents a row in the 2D array. You can access elements using their respective row and column indices. For instance, twoDArray[0][1]
would return 2
, the element in the first row and second column.
Output:
2
This method is straightforward and efficient for initializing a 2D array with known values. However, if you need to create a larger array dynamically, you may want to consider other methods.
Method 2: Using Nested Loops
If you’re looking to create a 2D array dynamically, especially when the size is not predetermined, using nested loops is a great approach. Here’s how you can implement this:
let rows = 3;
let cols = 3;
let twoDArray = new Array(rows);
for (let i = 0; i < rows; i++) {
twoDArray[i] = new Array(cols);
for (let j = 0; j < cols; j++) {
twoDArray[i][j] = i * cols + j + 1;
}
}
In this code snippet, we first define the number of rows and columns. We then create a new array for each row within a loop. The inner loop populates each element with a calculated value. This results in a filled 2D array where each element corresponds to its position in the grid.
Output:
[
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
This method provides flexibility, allowing you to create arrays of varying sizes and fill them with data based on your logic. It is particularly useful when working with user inputs or data fetched from APIs.
Method 3: Using Array.from()
Another elegant way to create a 2D array in JavaScript is by using the Array.from()
method. This method allows you to create a new array instance from an array-like or iterable object. Here’s how you can use it to create a 2D array:
let rows = 3;
let cols = 3;
let twoDArray = Array.from({ length: rows }, (_, i) =>
Array.from({ length: cols }, (_, j) => i * cols + j + 1)
);
In this example, we utilize Array.from()
twice. The outer call creates an array of the specified length for rows, while the inner call generates each row as an array of the specified length for columns. The arrow function calculates the value for each element based on its position.
Output:
[
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
This method is concise and leverages modern JavaScript features, making your code cleaner and more readable. It’s a great choice for developers who prefer functional programming styles.
Conclusion
In conclusion, understanding how to declare and manipulate 2D arrays in JavaScript is essential for any developer. Whether you choose to use array literals, nested loops, or the Array.from()
method, each approach has its advantages and can be utilized in different scenarios. As you continue to explore JavaScript, mastering these techniques will enhance your ability to manage complex data structures effectively. Happy coding!
FAQ
-
What is a 2D array in JavaScript?
A 2D array in JavaScript is an array that contains other arrays as its elements, allowing you to store data in a grid format. -
How can I access elements in a 2D array?
You can access elements using two indices: the first for the row and the second for the column, like this:array[rowIndex][columnIndex]
. -
Can I create a 2D array with different row lengths?
Yes, JavaScript allows you to create jagged arrays, where different rows can have varying lengths. -
What are some common use cases for 2D arrays?
Common use cases include representing matrices, game boards, and any data structure requiring a grid layout. -
Is it possible to iterate through a 2D array?
Yes, you can use nested loops to iterate through each row and column of a 2D array.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript